How to check if a string is a number in JavaScript
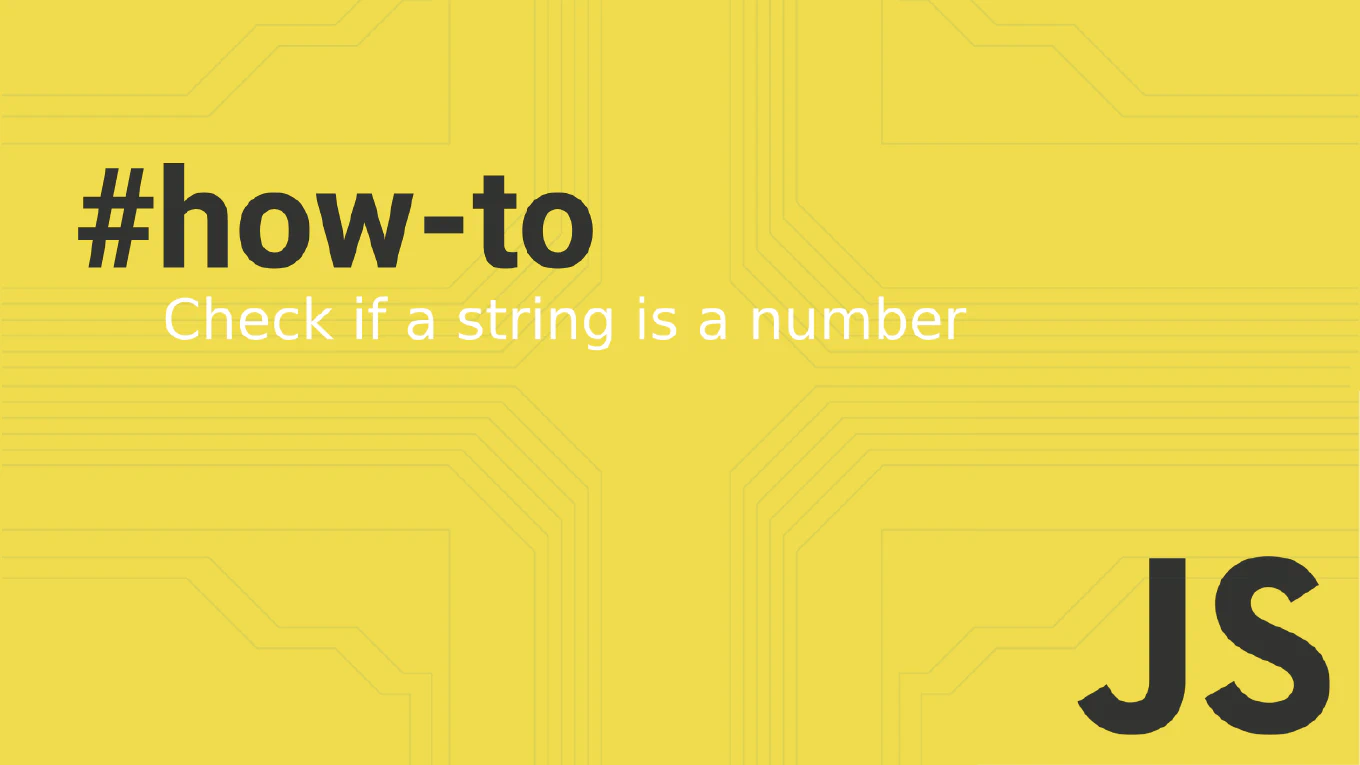
In the realm of web development, the versatility and power of JavaScript stand unchallenged. It is pivotal in adding interactivity to websites, working with data, and creating complex web applications. A common hurdle developers often stumble upon is determining whether a given piece of text—more formally, a string—represents a number. This validation is crucial for data processing, form submissions, and other scenarios where numeric input is expected. This comprehensive article explores the methods and best practices for checking if a string is a number in JavaScript, ensuring your code is robust, reliable, and ready for real-world usage.
The Challenge of String Validation
At first glance, checking if a string is numeric might seem straightforward. However, JavaScript’s dynamic typing and coercion can turn this simple task into a deceptive challenge. Incorrectly identifying a string as a numeric value could lead to bugs, incorrect data processing, or even security vulnerabilities. Hence, understanding the nuances of string validation in JavaScript is imperative for developers aiming to build secure and efficient web applications.
Fundamental Strategies for Numeric String Validation
Let’s dive into the core methods and strategies for validating numeric strings in JavaScript, highlighting their uses, benefits, and potential pitfalls.
Method 1: Using the isNaN
Function
The isNaN()
function is a global utility in JavaScript used to determine whether a provided value is NaN (Not-a-Number). While it may seem like the go-to solution, its behavior with strings requires careful consideration.
const value = '1234'
if (!Number.isNaN(value)) {
console.log('This string is numeric')
} else {
console.log('This string is not numeric')
}
Caveat: isNaN()
coerces the value to a number before testing. This means it considers numeric strings as numbers, potentially leading to false positives in cases where strict type-checking is needed.
Method 2: The Power of Regular Expressions
Regular expressions offer a more precise approach to validating numeric strings. By defining a pattern that matches numeric characters (optionally including decimal points and negative signs), developers can assert the numeric nature of a string with greater control.
const isNumeric = (string) => /^[+-]?\d+(\.\d+)?$/.test(string)
console.log(isNumeric('abcdef')) // Outputs: false
console.log(isNumeric('1')) // Outputs: true
console.log(isNumeric('1234')) // Outputs: true
console.log(isNumeric('1234.56')) // Outputs: true
console.log(isNumeric('-1234')) // Outputs: true
console.log(isNumeric('12345abcd')) // Outputs: false
console.log(isNumeric(1234)) // Outputs: true
console.log(isNumeric(1234n)) // Outputs: true
console.log(isNumeric('')) // Outputs: false
console.log(isNumeric(undefined)) // Outputs: false
console.log(isNumeric(null)) // Outputs: false
This method excels in scenarios requiring strict validation criteria, such as permitting or disallowing floating-point numbers and sign prefixes.
Method 3: Using the Number()
Function in JavaScript
JavaScript’s Number()
function is a handy tool for converting strings to numbers. It’s straightforward: if the string represents a valid number, Number()
converts and returns it. If not, you get NaN (Not-a-Number), signaling an unsuccessful conversion. Let’s break down its functionality with examples:
console.log(Number('12345')) // Outputs: 12345
console.log(Number('abcef')) // Outputs: NaN
console.log(Number('12345abc')) // Outputs: NaN
The Number()
function is essential for numeric conversions in JavaScript, distinguishing between convertible strings and those that lead to NaN. Its simplicity and clarity make it invaluable for processing and validating numeric data.
Method 4: Using the isFinite
Function
Introduced in ES6, Number.isFinite()
provides an elegant solution for identifying numeric values, including validating numeric strings when combined with type conversion.
const isNumeric = (string) => Number.isFinite(+string)
console.log(isNumeric('12345')) // Outputs: true
console.log(isNumeric('abcdef')) // Outputs: false
They are coercing the string to a number using the unary plus operator before the check mitigates the coercion pitfalls of isNaN()
, offering a more reliable check for numeric strings.
Method 5: Parsing Strings with parseFloat
and parseInt
The Number.parseFloat
and Number.parseInt
functions parse a string argument and return a floating-point number and an integer, respectively. To use them for validation, compare the original string with its parsed version.
const isNumeric = (string) => string == Number.parseFloat(string)
console.log(isNumeric('12345.00')) // Outputs: true
console.log(isNumeric('12345abc')) // Outputs: false
This method is beneficial when validating and using the numeric value simultaneously, ensuring it conforms to the expected format.
Advanced Tips and Considerations
While the abovementioned techniques provide a solid foundation, mastering numeric string validation in JavaScript involves attention to edge cases and advanced scenarios. Please consider the implications of leading zeros, scientific notation, or locale-specific number formats in your validation logic to enhance its accuracy and flexibility.
Wrapping Up: The Takeaway on Numeric String Validation in JavaScript
JavaScript offers multiple pathways to validate if a string is numeric, each with its unique advantages and considerations. By carefully selecting the most appropriate method based on your specific requirements—strict pattern matching with regular expressions, the robustness of Number.isFinite
, or the straightforward approach of parsing—the integrity of your data processing routines can be significantly bolstered.
Remember, the key to successful numeric string validation lies in understanding the capabilities and the limitations of JavaScript’s type coercion and parsing behaviors. Armed with this knowledge, you’re now better equipped to tackle the challenges of working with numeric strings in your JavaScript projects, enhancing your web applications’ security and reliability.
We hope this guide has given you valuable insights into how to validate numeric strings in JavaScript. You can go deeper into each method, experiment with code examples, and apply these strategies in your development endeavors for more robust and error-free applications.