How to sort an array of objects by string property value in JavaScript
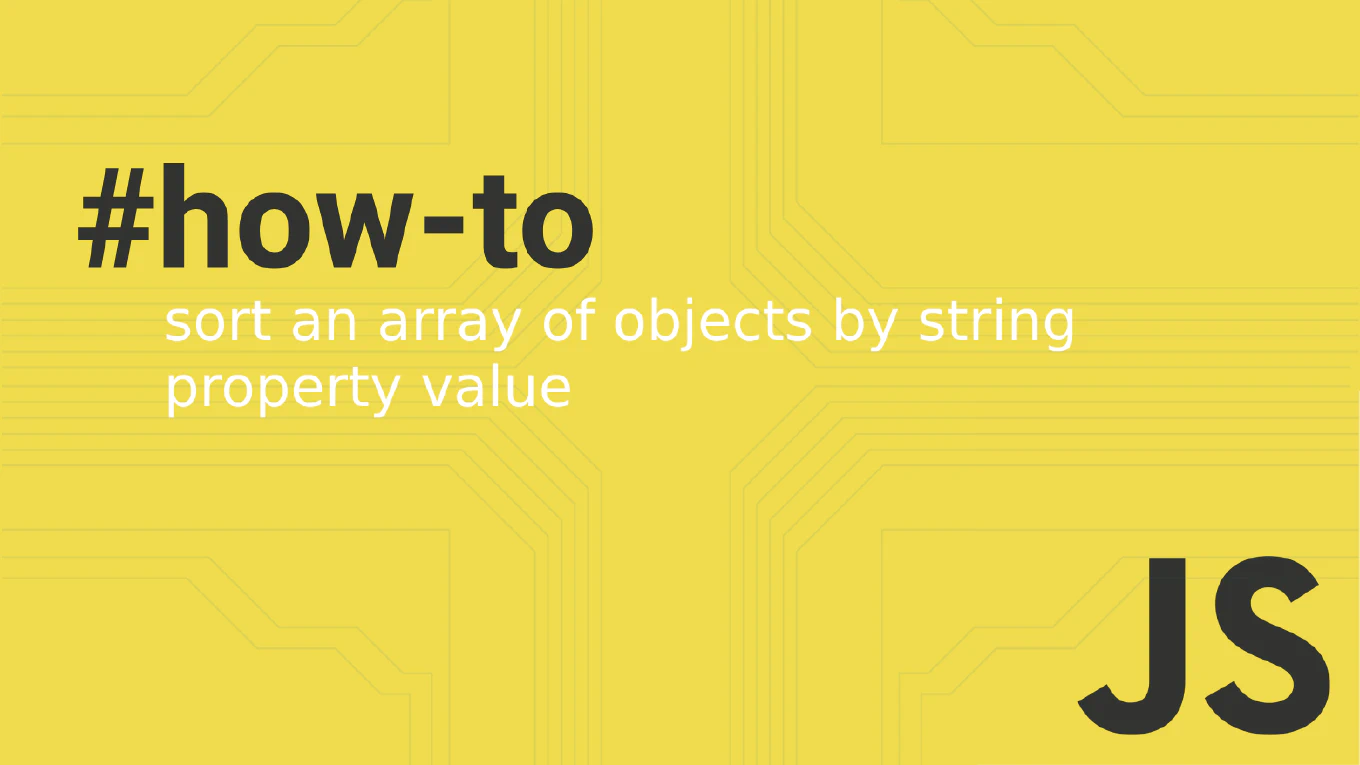
Sorting an array of objects by a string property value is a common task in JavaScript development. Whether you’re working with user data, product listings, or any other complex data structures, understanding how to sort arrays effectively can enhance your applications’ functionality and user experience. In this article, we’ll explore various methods to sort an array of objects by a string property value in JavaScript, utilizing the powerful sort
method and custom comparison functions.
Understanding the sort
Method
JavaScript provides the sort
method as a built-in array method for sorting an array’s elements. By default, the sort
method sorts array elements as strings in ascending order, which might not always be suitable for sorting objects based on specific property values. A comparison function is essential to achieve more control over the sorting process, especially when dealing with objects.
Using a Compare Function
A compare function is a crucial part of the sorting mechanism when you need to sort an array of objects based on a particular key value. The compare function takes two parameters, typically referred to as a
and b
, representing two array elements. This function should return a
negative value if a should come before b
, a positive value if a
should come after b
, or zero if considered equal in the sort order.
Here’s a basic example of using a compare function to sort an array of objects by a string property:
const users = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
]
const sortedUsers = users.sort((a, b) => {
if (a.name < b.name) return -1
if (a.name > b.name) return 1
return 0
})
console.log(sortedUsers)
In this example, the sort
method uses a comparison function that compares the name
property of each object, resulting in a sorted array in ascending order based on the users’ names.
Creating a Custom Comparison Function
Creating a custom comparison function is beneficial for more flexibility, especially when dealing with different data types or sorting orders. A custom comparison function allows you to define specific sorting logic tailored to your application’s needs.
Sorting in Ascending and Descending Order
You can sort an array in descending order by adjusting the comparison function to invert the return values.:
const sortedUsersDesc = users.sort((a, b) => {
if (a.name < b.name) return 1
if (a.name > b.name) return -1
return 0
})
console.log(sortedUsersDesc)
This adjustment ensures the array is sorted in descending order based on the name
property.
Handling Case Sensitivity
String comparison in JavaScript is case-sensitive by default. To perform a case-insensitive sort, you can convert the string values to a common case (either lowercase or uppercase) before comparison:
const sortedUsersCaseInsensitive = users.sort((a, b) => {
const nameA = a.name.toLowerCase()
const nameB = b.name.toLowerCase()
if (nameA < nameB) return -1
if (nameA > nameB) return 1
return 0
})
console.log(sortedUsersCaseInsensitive)
By converting both nameA
and nameB
to lowercase, the sort becomes case-insensitive, ensuring a more consistent sorting order regardless of the original casing.
Sorting Complex Data Structures
When dealing with more complex data structures, such as objects containing date strings or numeric values, the comparison function can be enhanced to handle different data types appropriately.
Sorting by Date Strings
If your array of objects includes date strings, you can sort them by converting the date strings to Date
objects within the comparison function:
const events = [
{ event: 'Conference', date: '2024-05-20' },
{ event: 'Meeting', date: '2023-12-15' },
{ event: 'Workshop', date: '2024-01-10' }
]
const sortedEvents = events.sort((a, b) => new Date(a.date) - new Date(b.date))
console.log(sortedEvents)
This approach ensures the array is sorted chronologically based on the date
property.
Leveraging Utility Functions for Dynamic Sorting
Creating utility functions for sorting can streamline the process, especially when you need to sort by different properties dynamically. Here’s an example of a dynamic sort function that can sort an array based on any specified key:
const dynamicSort = (key, order = 'asc') => {
return (a, b) => {
const valA = a[key].toLowerCase()
const valB = b[key].toLowerCase()
if (valA < valB) return order === 'asc' ? -1 : 1
if (valA > valB) return order === 'asc' ? 1 : -1
return 0
}
}
const sortedUsersDynamic = users.sort(dynamicSort('name', 'desc'))
console.log(sortedUsersDynamic)
This utility function, dynamicSort
, takes a key
and an optional order
parameter, allowing you to sort the array in ascending or descending order based on the specified property.
Conclusion
Sorting JavaScript array of objects by a string property value in JavaScript is a fundamental skill that can significantly enhance data manipulation and presentation in your applications. By utilizing the sort
method with custom comparison functions, you can achieve precise and efficient sorting tailored to your specific requirements. Whether you’re handling simple datasets or complex data structures, mastering these techniques will empower you to manage and display data effectively.
Additional Tips
-
Sort Stability: Modern JavaScript engines implement stable sorting, meaning elements with equal keys retain their original order. This is useful when sorting by multiple properties sequentially.
-
Performance Considerations: Consider the performance implications of your comparison functions for large arrays. Efficient functions can significantly reduce sorting time.
-
Immutable Sorting: If you need to preserve the original array, use methods like
slice
to create a copy before sorting:const sortedCopy = users.slice().sort((a, b) => a.name.localeCompare(b.name))
By following these practices, you can ensure that your array sorting logic is robust and efficient.