Mastering Inline Styles in React.js: Enhancing Your Components with Style
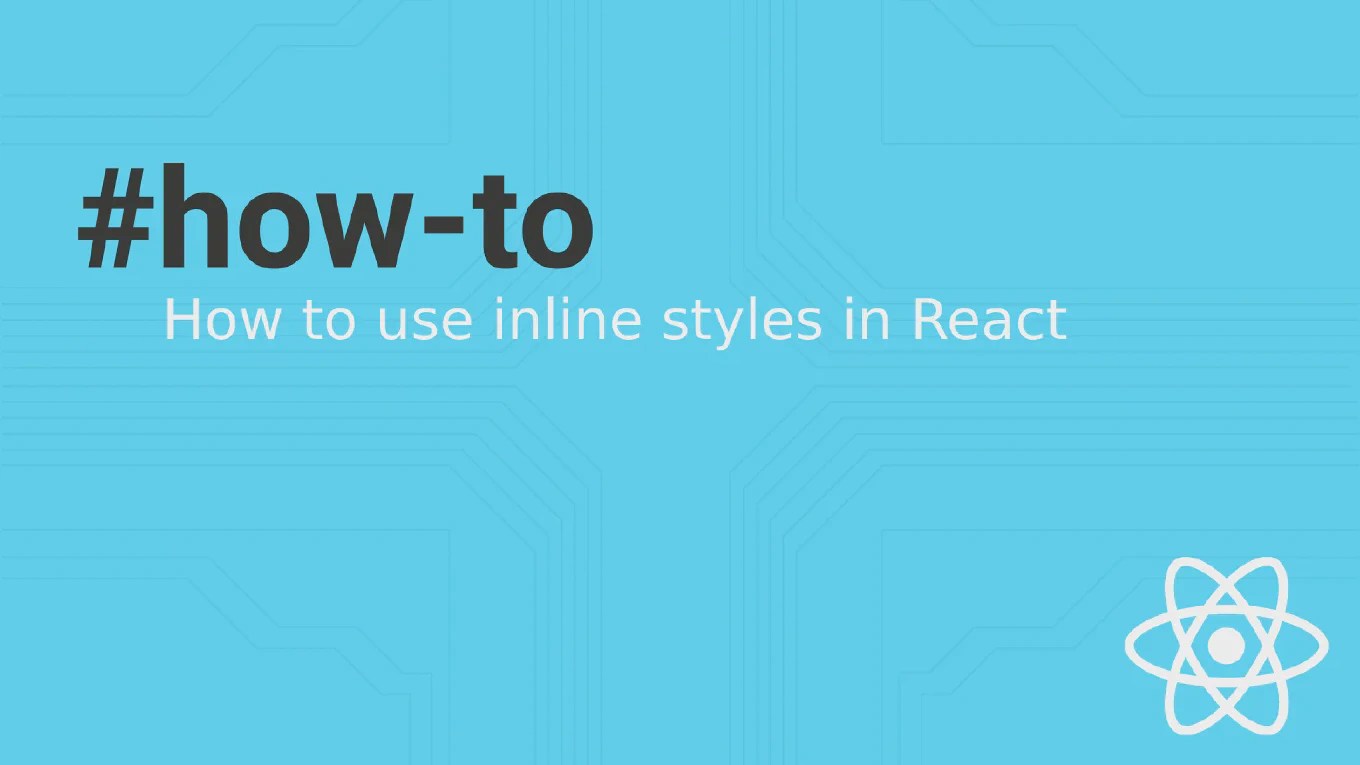
Are you looking to refine your React.js components with a dash of styling, but find yourself tangled in a web of CSS files? Fear not! Inline styles in React can be a powerful tool for developers to directly insert styling properties into their components. Here, we delve into the art of using react inline style, answering critical questions and unveiling the best practices to maximize the potential of your React.js applications. Whether you’re painting your first component or tweaking an existing masterpiece, this guide will illuminate the path to impeccable styling within your React projects.
The Place of Inline Styles in React.js
The juxtaposition of inline styles within React can at first appear as a conflict against the natural order of cascading stylesheets. However, understanding the use and versatility of inline styles helps unveil their strategic importance. By applying styles directly on a per-element basis, developers gain granular control, swift deployment of component-specific styles, and sidestep the complexities of managing numerous stylesheets.
But before you set sail on this technique, let’s clarify what it entails:
Can I use inline style in React?
Certainly! Inline styles are not only possible in React, but they also come with the framework’s special enhancements. React makes use of a style attribute that accepts a JavaScript object with camelCased properties rather than a string, offering a more dynamic and expressive way to apply CSS rules.
How do you use inline style variable in React?
Using an inline style variable in React involves defining an object that contains CSS properties and their corresponding values. Here’s a simple example:
const componentStyle = {
color: 'blue',
backgroundColor: 'lightgrey',
padding: '10px',
borderRadius: '5px'
}
function MyStyledComponent() {
return <div style={componentStyle}>Stylish Div</div>
}
Are inline styles okay?
Inline styles can be a quick and hassle-free method for adding styles to your components, especially for simple projects or prototype designs. They are also useful when you need a style that is computed at runtime. However, they should be used judiciously as they can quickly clutter your components, override your stylesheets, and become a challenge to manage for larger applications.
Do inline styles override stylesheets in React?
Yes, inline styles have the highest specificity when it comes to CSS and therefore will override styles set in external stylesheets, unless those styles are marked with !important. It’s crucial to be mindful of this to prevent unintentional styling results.
Composing Styles with Precision
Navigating through the realm of styling in React can be likened to composing a symphony; each note must be placed with intention and understanding. Let’s explore how to compose inline styles with precision.
Organizing Your Styles
Organizing inline styles involves creating a structured approach:
Define your style objects near the top of your component file for clarity. Use descriptive names for your style objects to reflect their purpose. Group related styles together for maintainability.
Consider the following example:
const headerStyle = {
textAlign: 'center',
fontSize: '24px',
fontWeight: 'bold'
}
const buttonStyle = {
padding: '15px 30px',
border: 'none',
borderRadius: '5px',
cursor: 'pointer'
}
function PageHeader() {
return (
<header style={headerStyle}>
<h1>Welcome to My Page</h1>
<button style={buttonStyle}>Click Me</button>
</header>
)
}
Crafting a Story with Styles
When using inline styles, you craft a visual narrative for your users. Think of each style object as a character in your story. As you write your styles, remember that every visual element should serve a purpose and contribute to the user experience.
A Practical Example
Imagine you’re creating a notification component that needs to change its appearance based on its type prop, which could be “success”, “info”, “warning”, or “error”. You could use inline styles like this:
function Notification({ type, message }) {
const sharedStyle = {
padding: '10px 20px',
margin: '10px 0',
border: '1px solid transparent',
borderRadius: '4px',
textAlign: 'center',
color: 'white'
}
const typeStyles = {
success: { ...sharedStyle, backgroundColor: 'green' },
info: { ...sharedStyle, backgroundColor: 'blue' },
warning: { ...sharedStyle, backgroundColor: 'orange' },
error: { ...sharedStyle, backgroundColor: 'red' }
}
return (
<div style={typeStyles[type]}>
{message}
</div>
)
}
In this example, dynamic styling is achieved efficiently through combining a base style with type-specific styles to tell the right visual story for each notification.
Performance and Pitfalls: When to Use Inline Styles
It’s vital to remember that with great power comes great responsibility. Inline styles should ideally be used sparingly due to potential maintenance issues and performance concerns, particularly with large-scale applications or complex dynamic styles. Opt instead for stylesheets or CSS-in-JS libraries like Styled-Components or Emotion for most styling needs.
However, in situations where you need to:
- Apply styles that are computed based on props or state at runtime.
- Iterate over items and apply unique styles based on data.
- Style a component that is rarely used or doesn’t require theme consistency.
Inline styles might be the best approach.
Essential Takeaway
Inline styles in React offer a convenient method to apply styles directly to components, offering a straightforward approach to component-specific styling. However, keep an eye out for the trade-offs and use them wisely to ensure your project remains manageable and performant.
Remember, the journey to master styling in React is not a sprint but a marathon. Adapt your styling approach to your project’s requirements, maintain an organized pattern, and most importantly, continually refine your strategy for writing efficient and effective inline styles.
As you incorporate these practices into your development workflow, you’ll not only enhance your applications with visually compelling components but also improve your skills as a React developer.