How to Redirect to a New URL Using JavaScript Redirect Techniques
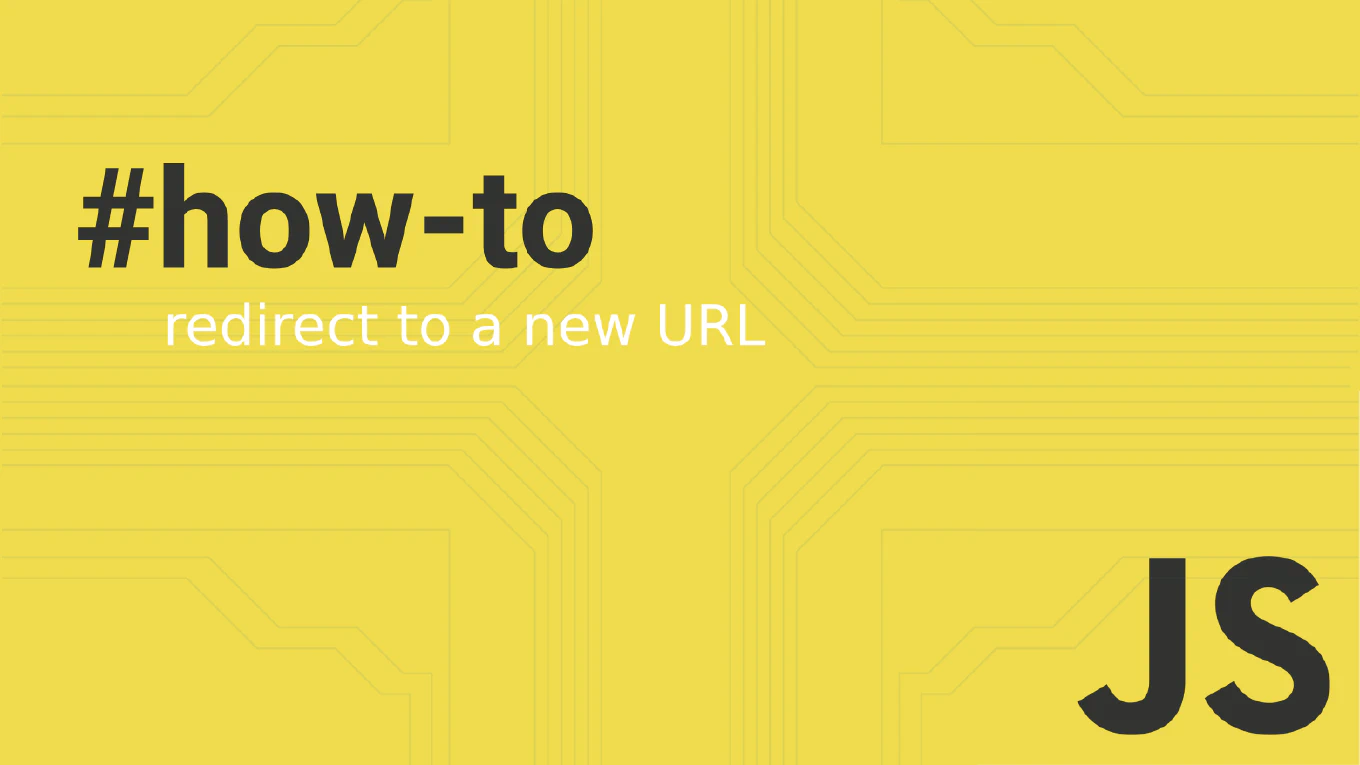
Redirecting users to a new URL using JavaScript is a common and useful practice for web developers. Whether you’re handling successful logins, navigating users after actions, or managing SEO implications, understanding how JavaScript redirects work is crucial. In this guide, you’ll learn the best practices for creating redirects, their implications for SEO performance, and when to choose JavaScript over server-side methods.
How to Achieve Perfectly Rounded Corners in CSS
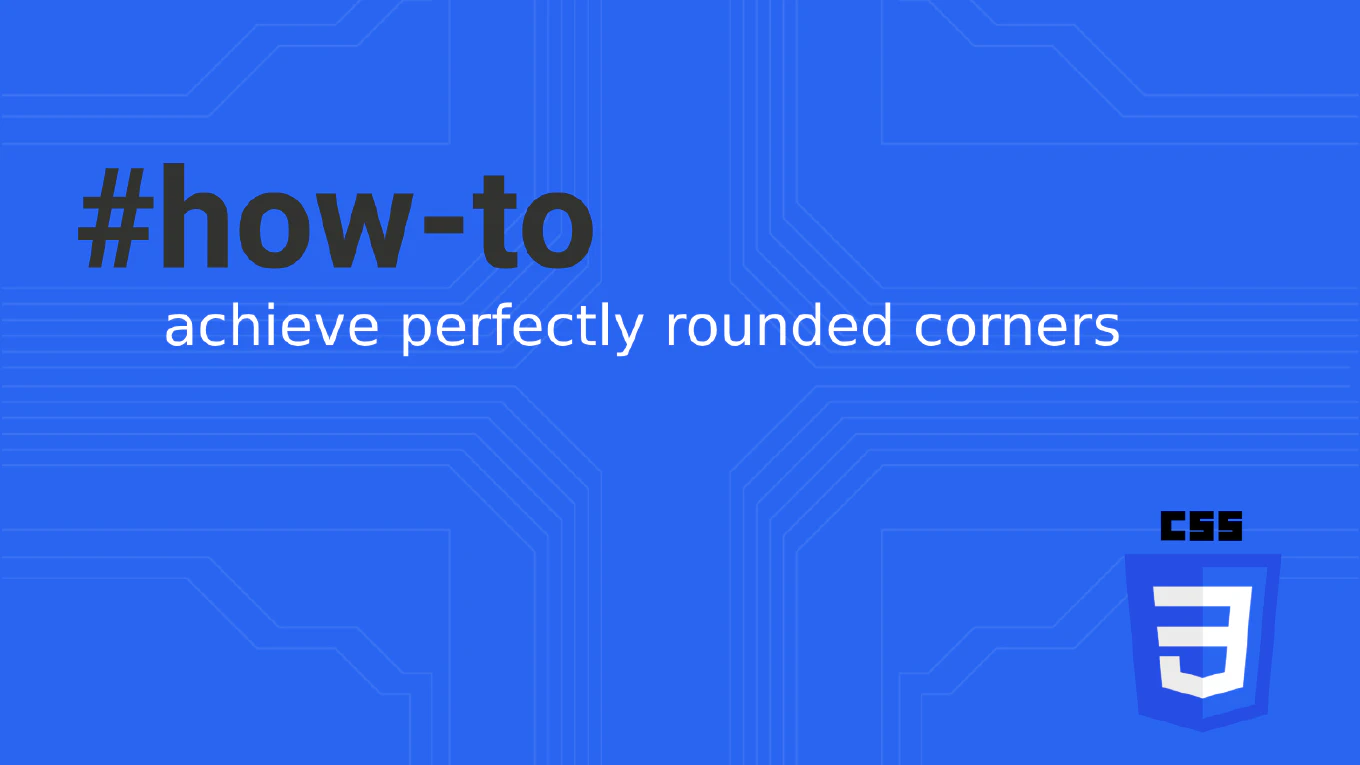
Rounding the corners of an element is a fundamental way to add visual appeal and flexibility to web pages. Whether you want subtle rounded corners, a circle, or even elliptical corners, mastering the border radius property is a key step toward creating modern, user-friendly interfaces. In this post, you will learn how to use the CSS border radius property, explore best practices for top left and bottom corners, handle right bottom left edges, and even add a box shadow or drop shadow for extra flair. By the end, you will be able to style each corner effectively, apply logical properties for both LTR and RTL layouts, and bring your web designs to life.
How to Add a Tab in HTML
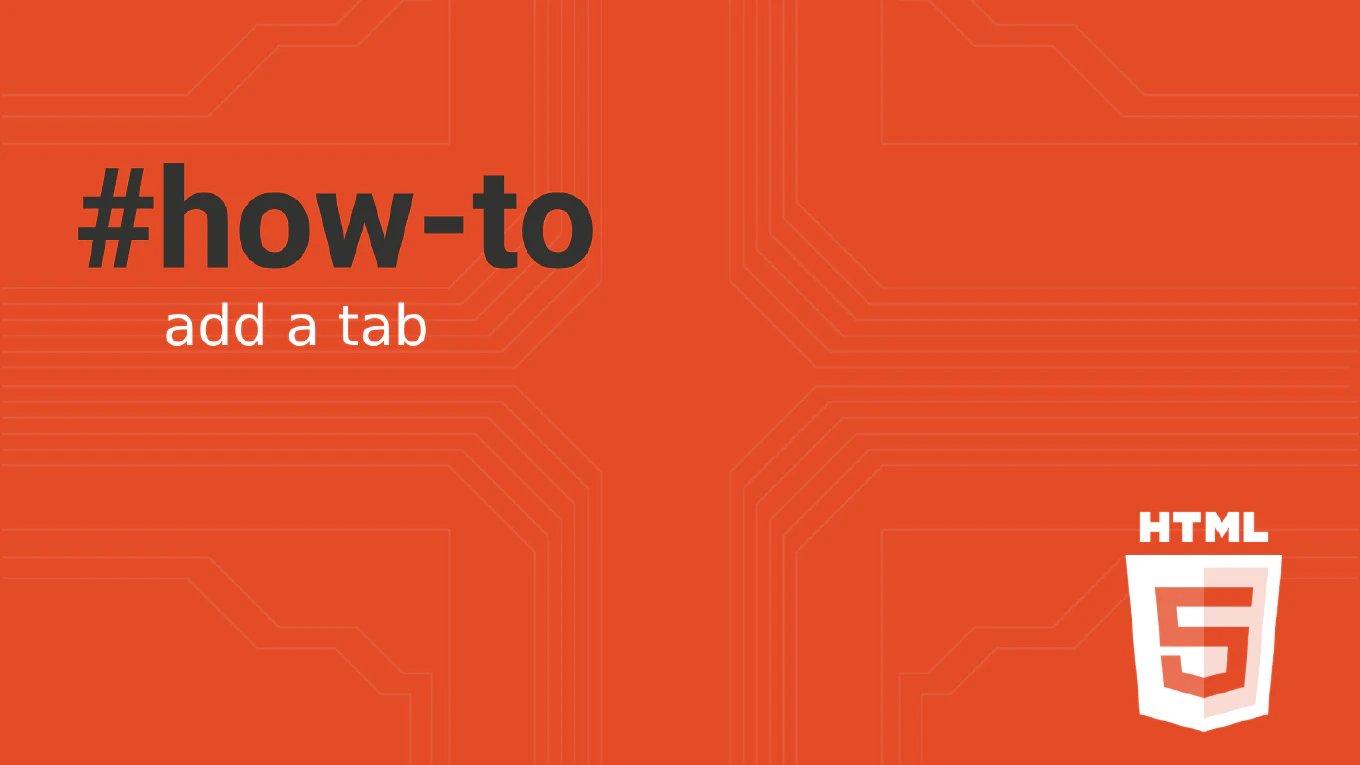
Adding a tab in HTML is a common challenge for developers who want to control spacing and layout on their web pages. Since many browsers and editors interpret the tab key differently, it’s crucial to understand several ways to produce a consistent horizontal tab effect. In this article, you’ll learn key methods for creating a tab character in HTML, explore the best CSS properties to manage spacing, and see how to maintain accessibility for all users. By the end, you’ll have a clear solution for implementing tab space without relying on multiple non breaking spaces.
How to Open Link in a New Tab in HTML?
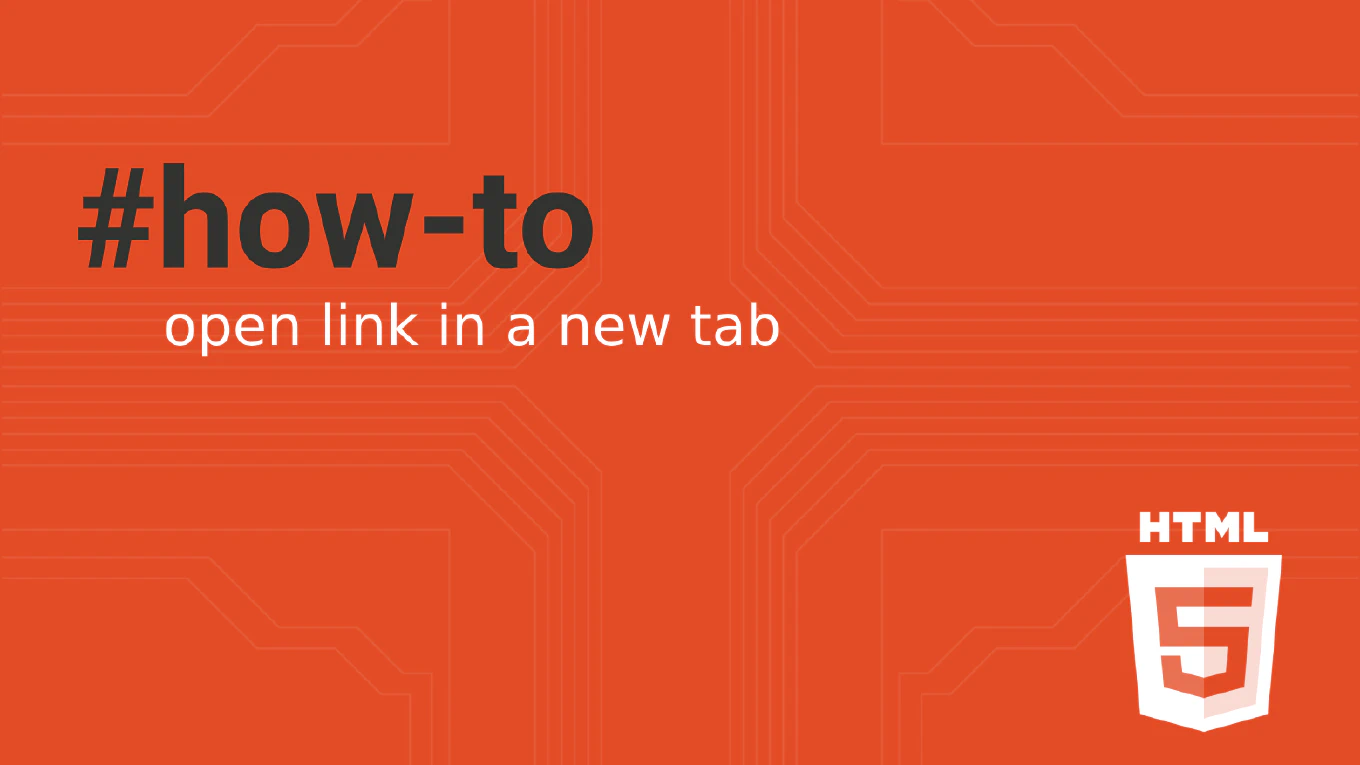
Opening a link in a new tab is a fundamental skill for anyone building modern websites or web applications. By using specific HTML attributes, you can help your users effortlessly view external content or switch between related pages without losing their place on the original page. In this article, you will learn why the target
attribute is crucial, how to properly handle potential security concerns, and the best techniques to open the linked document in a separate browser window or tab. Read on to discover how you can enhance user experience and stay in control of your own site’s navigation flow.
How to Clone an Object in JavaScript
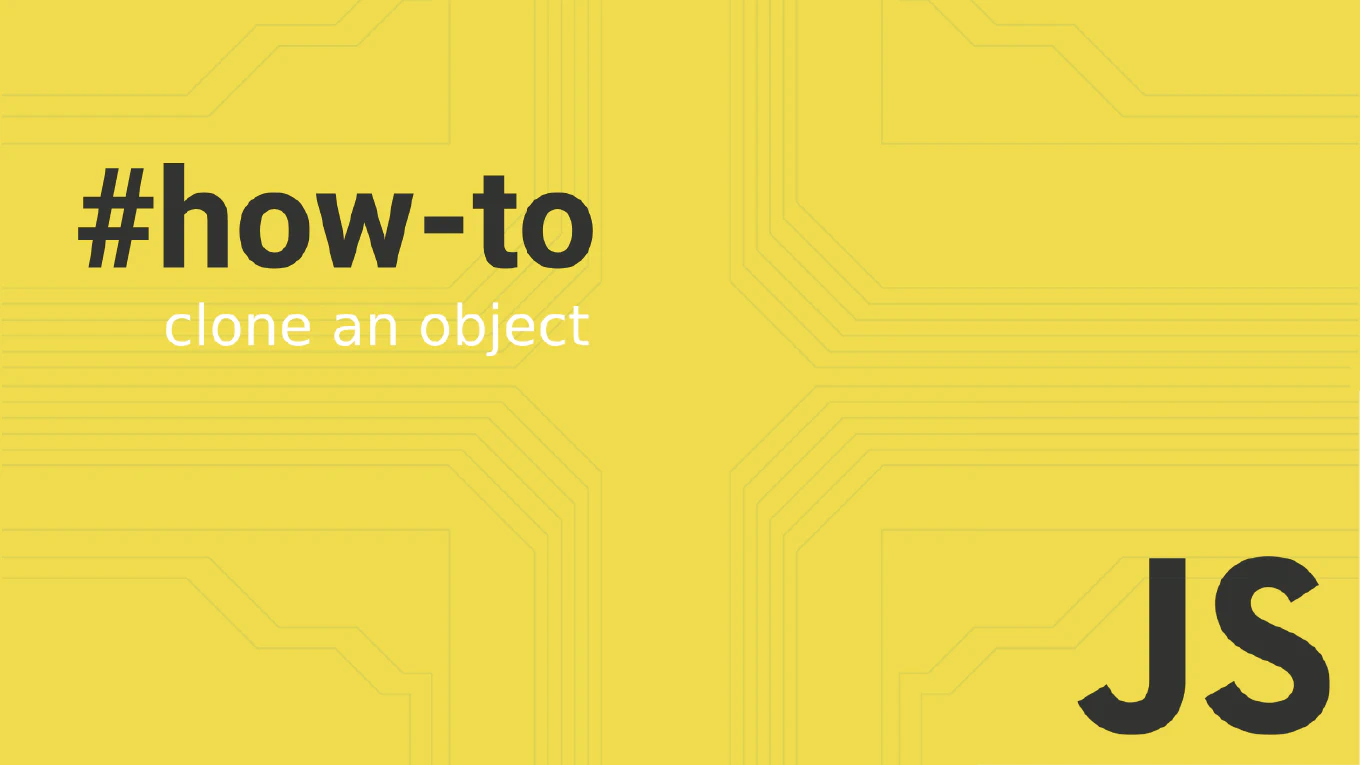
Cloning or duplicating objects in JavaScript is a common task for developers who need to avoid unintended side effects when sharing data. If you simply use the assignment operator to reference one object from another, you risk mutating the same object instead of creating a new object. By learning how to copy an object correctly, you can keep your code clean, ensure data integrity, and handle everything from simple object literals to more advanced scenarios such as nested objects. In this article, you’ll discover practical methods for copy object javascript, including both shallow copy and deep copy techniques, and see how to deal with edge cases like circular references or an undefined value.
How to Fix “Sass @import Rules Are Deprecated and Will Be Removed in Dart Sass 3.0.0.”
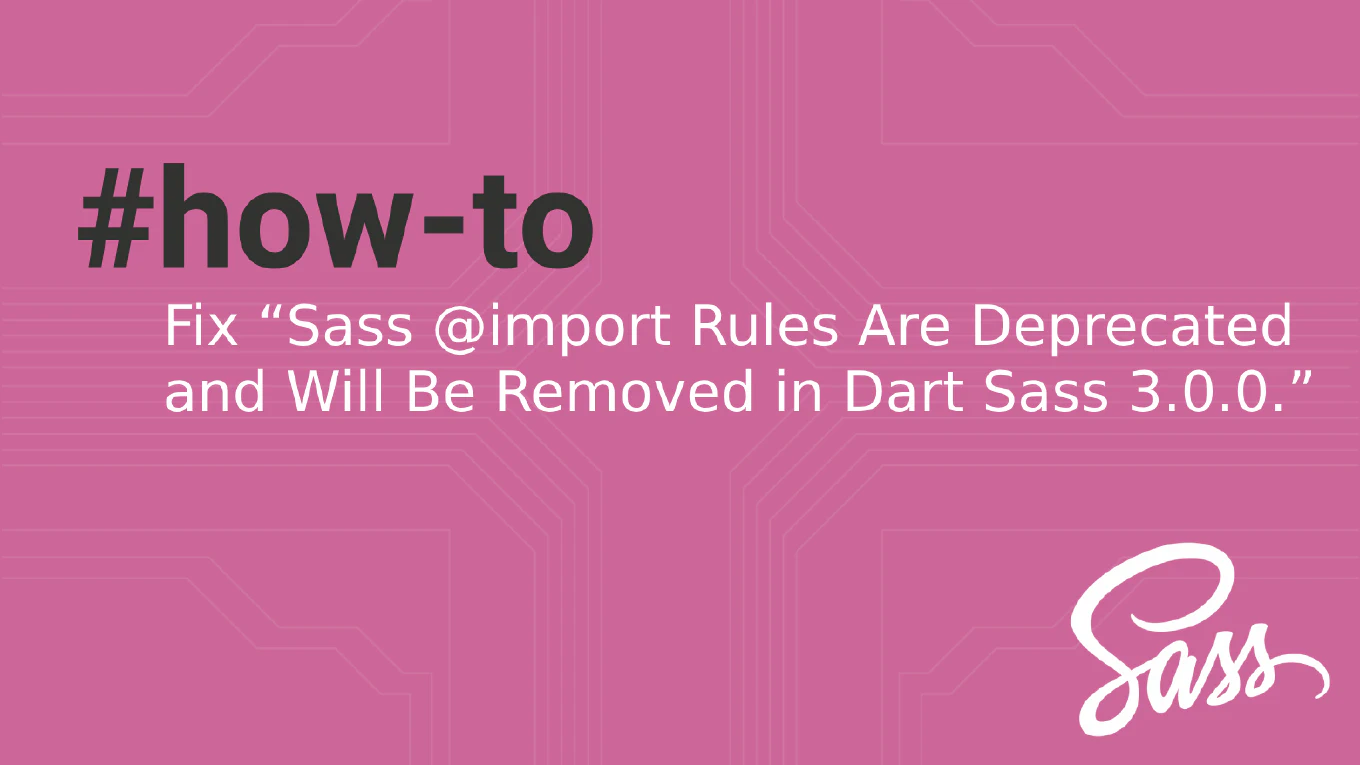
For years, the @import
rule has been the go-to approach for handling modular Sass code. However, the Sass team announced the deprecation of @import
in favor of the newer module system. If your build process or existing codebase shows a warning—“Sass @import rules are deprecated and will be removed in Dart Sass 3.0.0.”—it’s time to switch to the recommended @use
or @forward
directives. In this post, you’ll learn why the Sass team introduced this change, the best practices for migrating your code, and how to ensure your workflow remains smooth and maintainable.
How to Remove Elements from a JavaScript Array
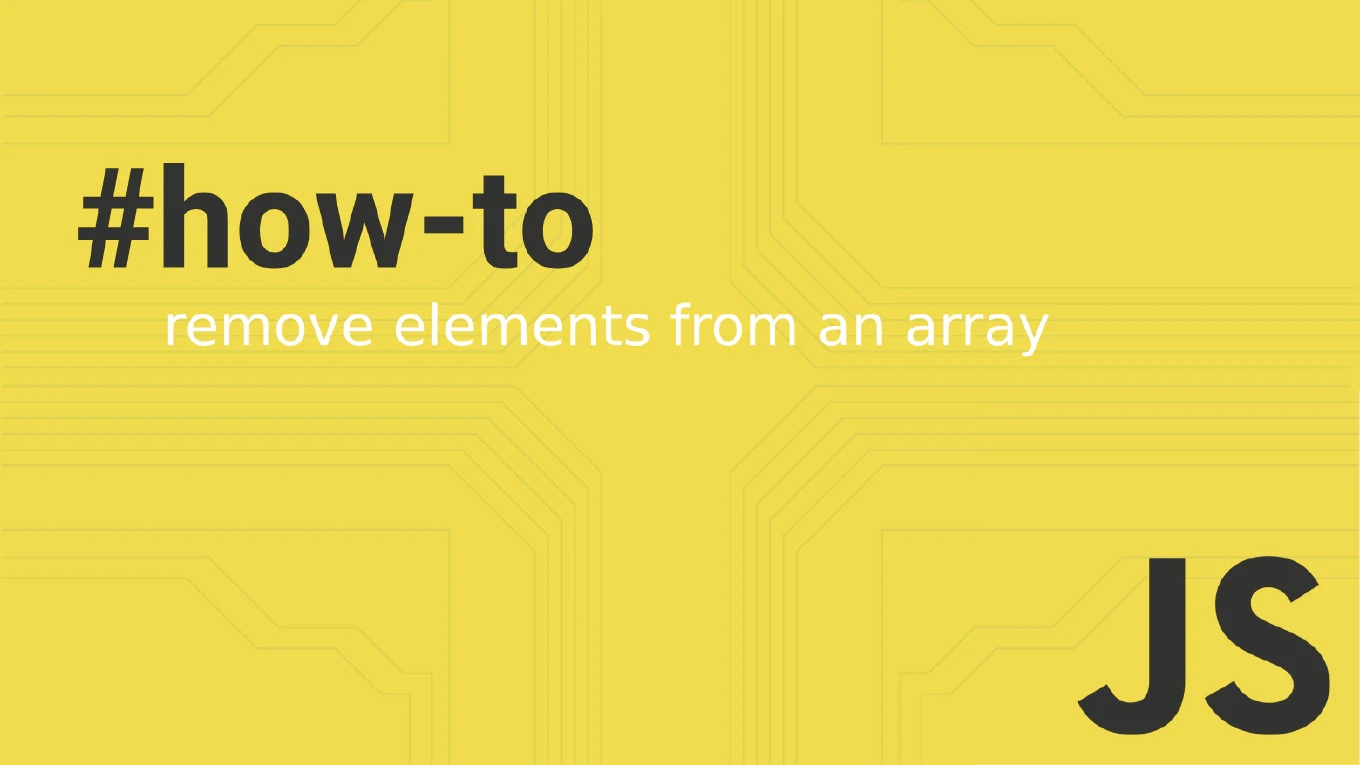
Removing elements from an array in JavaScript is a fundamental skill every developer should master. Whether you need to remove the first element, remove the last element, or filter out unwanted elements based on a specified value, understanding multiple techniques to remove array elements ensures your code remains clean and efficient. In this post, we will explore a few methods to remove element from array in javascript, explain how they work on the original array or when they create a new array, and show you the potential pitfalls.
What is the Difference Between Null and Undefined in JavaScript
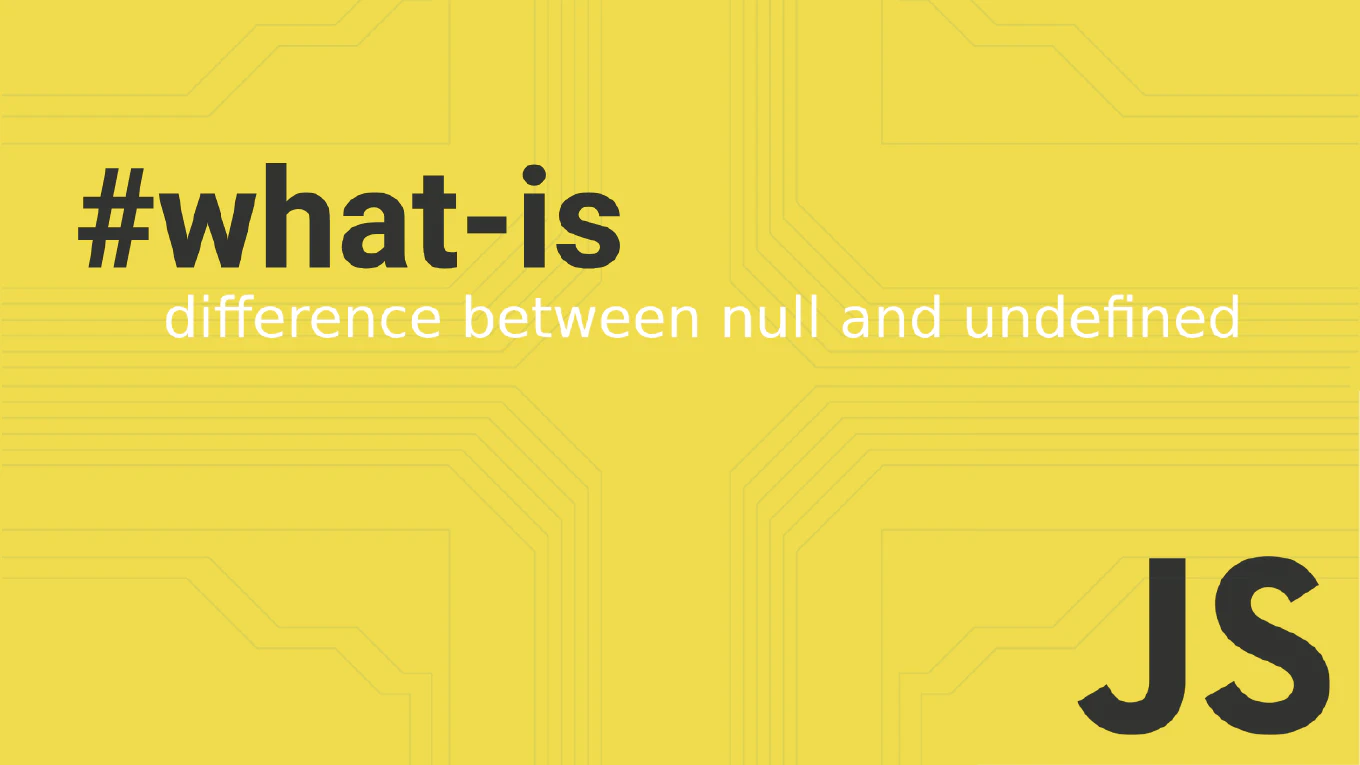
If you’ve ever wondered about the differences in JavaScript null
vs undefined
, read on. In the JavaScript programming language, the difference between null
and undefined
can seem confusing to many developers. Although both represent the absence of a meaningful value, they are distinct types in JavaScript with their own inner workings. Learning how to handle null/undefined
scenarios is crucial because these two values frequently appear in everyday coding tasks. By understanding undefined
in JavaScript and null
in real-world cases, you can avoid unexpected bugs, properly manage data types, and maintain cleaner code. This article will walk you through the subtle difference between null
and undefined
, and offer best practices for handling them.
How to Manage Date and Time in Specific Timezones Using JavaScript
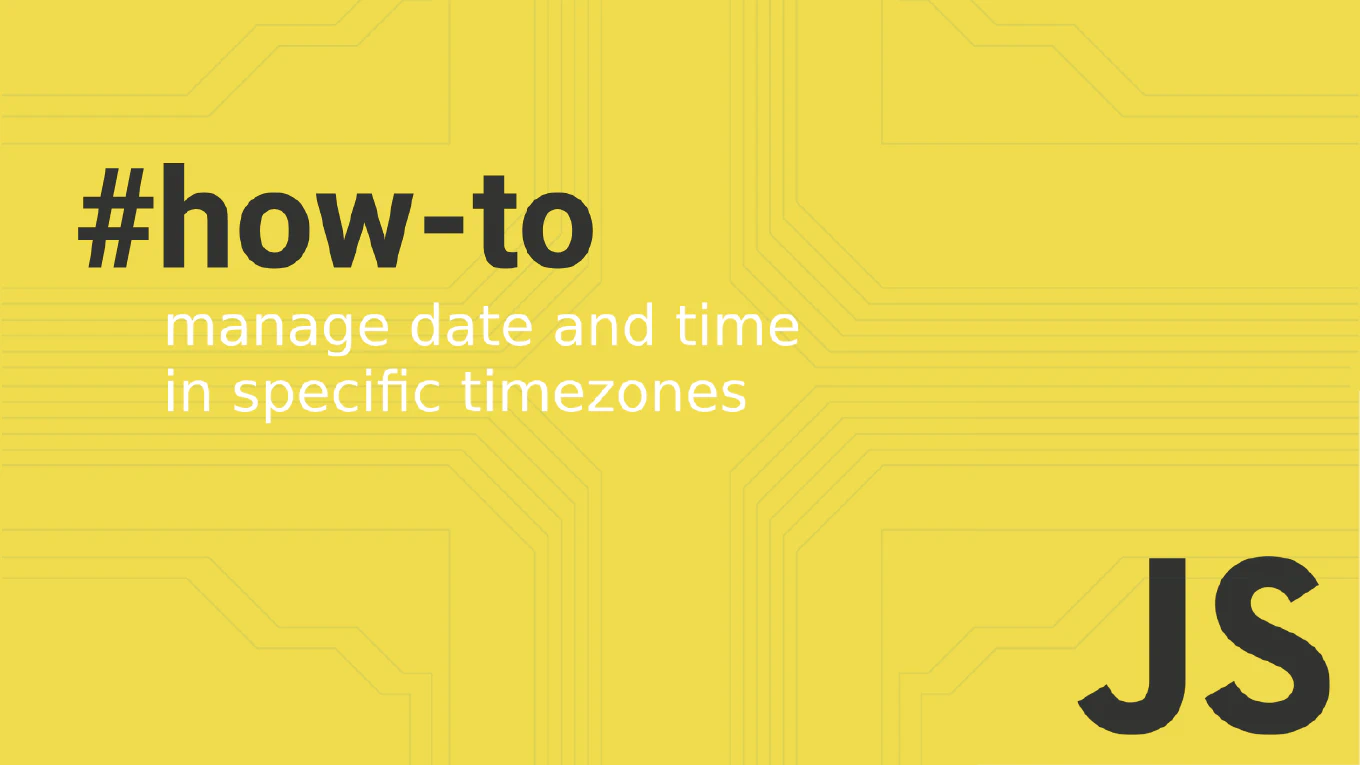
Handling date and time across different timezones is a common yet intricate task for JavaScript developers. Whether you’re working on global applications or time-sensitive services, precise date and time manipulation in specific timezones is essential. JavaScript provides native methods and modern APIs to simplify this task, but challenges like daylight saving time, timezone offsets, and formatting can still cause issues.
How to sort an array of objects by string property value in JavaScript
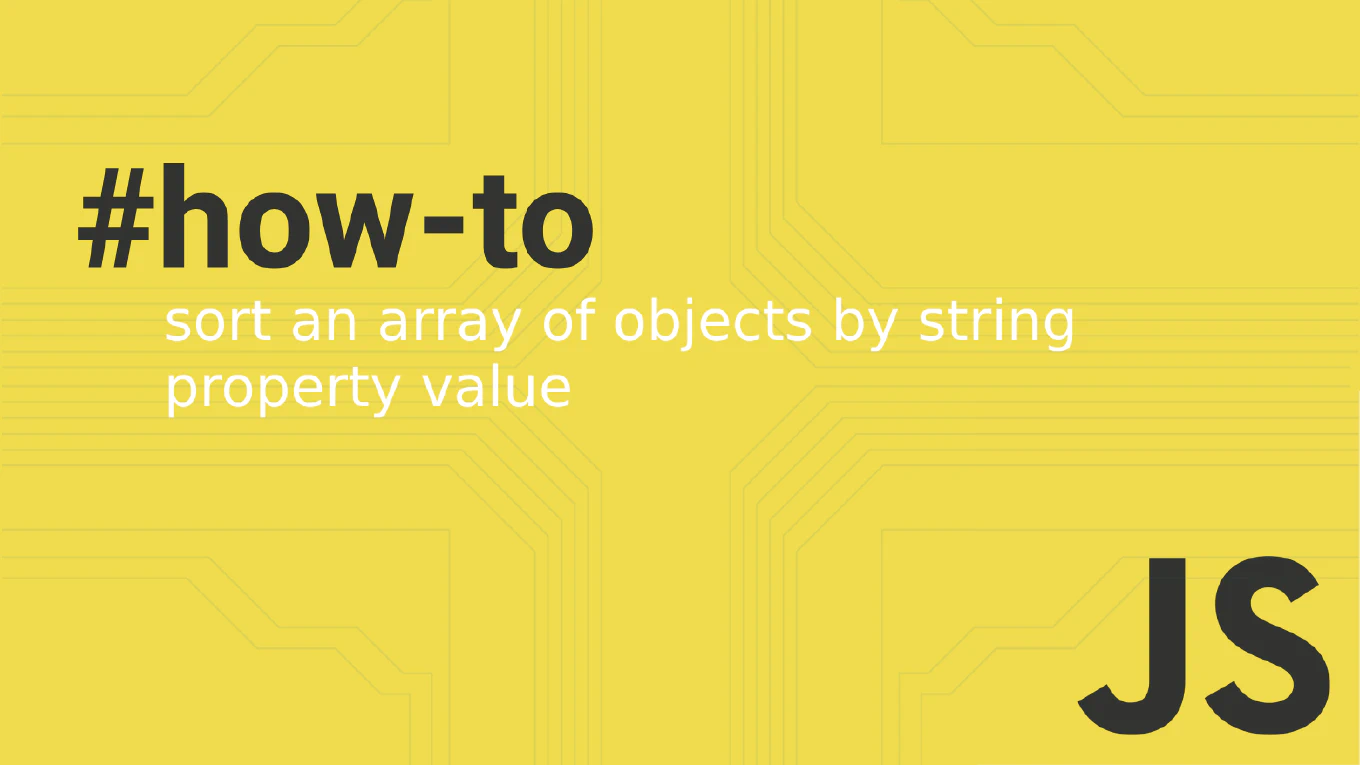
Sorting an array of objects by a specific property is a common requirement in JavaScript, especially when dealing with complex data structures in real-world applications. In this article, we’ll explore how to sort an array of objects by string values using JavaScript’s sort
method. By the end of this guide, you’ll understand how to implement a custom comparison function and sort arrays efficiently.