Mastering the Spread Operator (`...`) in React.js
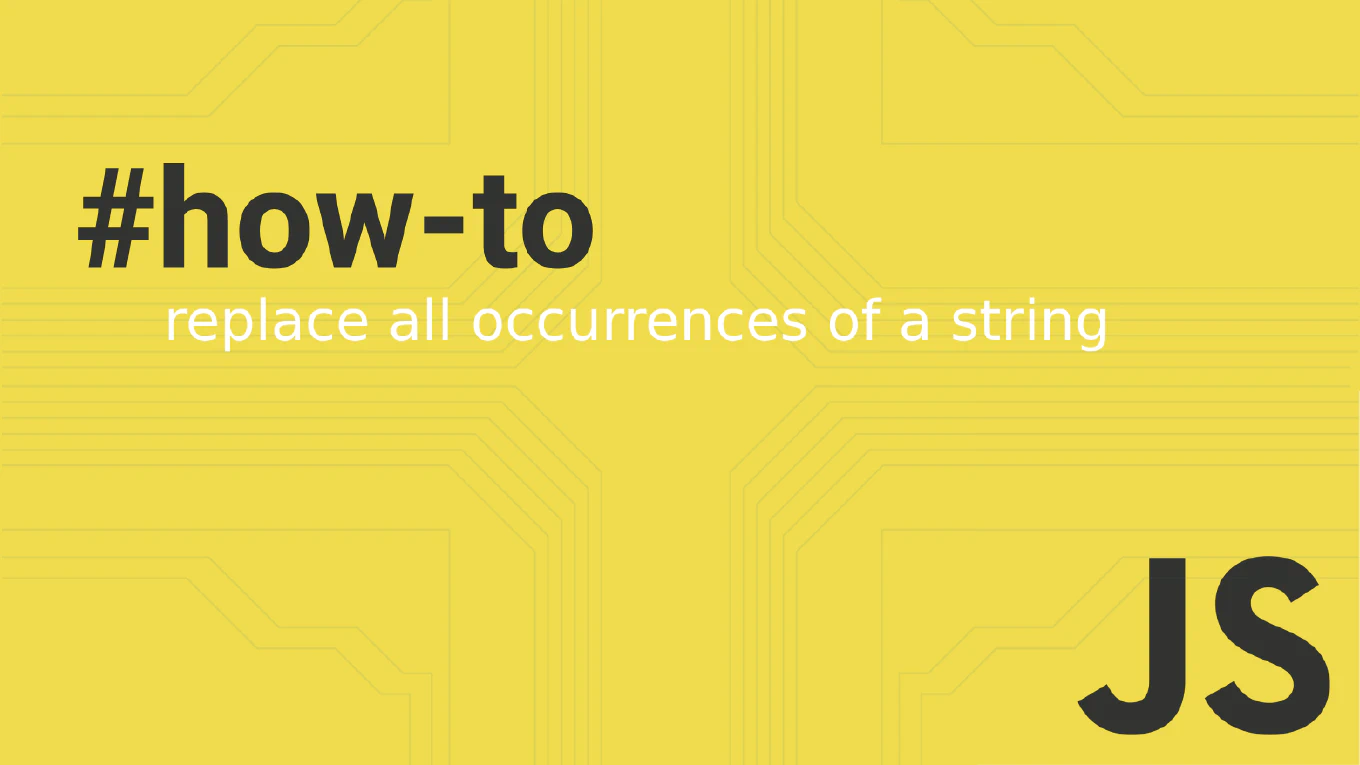
Introduction
In React.js, the three dots (...
), commonly known as the spread operator, are a powerful tool for managing state, props, and arrays within your applications. The spread syntax simplifies many common tasks, such as merging objects, copying arrays, and passing props to components. Understanding how to effectively use the spread operator is crucial for building efficient and maintainable React components.
Using the JavaScript Spread Operator in React
Passing Props to React Components
One of the most common uses of the spread operator in React is when passing props to child components. Instead of manually specifying each prop, you can use the spread syntax to pass all properties of a props object at once.
Example: Passing Props Without the Spread Operator
function MyButton() {
return <button type='button' className='btn-primary' disabled={false}>Click Me</button>
}
In this version, every prop is explicitly passed to the button
component. This approach can become cumbersome and error-prone, especially as the number of props increases.
Example: Passing Props with the Spread Operator
const props = { type: 'button', className: 'btn-primary', disabled: false }
function MyButton() {
return <button {...props}>Click Me</button>
}
In this first example using the spread operator, the spread syntax is used to pass all properties from the props
object to the button
element. This approach is particularly useful when dealing with dynamic props or when the props object contains multiple elements that need to be passed down to a child component.
By using the spread operator, you can avoid repetitive code and ensure that your components remain easy to manage, even as the number of props grows.
Merging State in React Components
React applications often require managing complex state objects. The spread operator allows you to easily merge multiple objects into a new object, which is especially useful when updating state in React components.
Example: Merging State Objects
const [user, setUser] = useState({ name: 'Alice', age: 25 })
function updateUser(newDetails) {
setUser({ ...user, ...newDetails })
}
In this example, the spread operator is used to merge newDetails
into the existing user
state. By using the spread syntax, only the properties in newDetails
are updated, while the other properties in the existing object remain unchanged. This method is particularly effective when dealing with nested objects or when updating specific key value pairs in the state.
Managing Arrays in React State
Copying and Updating Arrays
When working with arrays in React state, the spread operator simplifies the process of creating a new array that includes updates or additions without mutating the existing array.
Example: Adding Elements to an Array in State
const [items, setItems] = useState(['item1', 'item2'])
function addItem(newItem) {
setItems([...items, newItem])
}
In this example, the spread operator is used to create a new array that includes all elements from the existing array plus newItem
. This ensures that the original array remains unchanged, which is a core principle in React’s state management.
Concatenating Arrays
The spread operator also makes it easy to concatenate arrays in React.
const array1 = [1, 2, 3]
const array2 = [4, 5, 6]
const mergedArray = [...array1, ...array2]
Here, array1
and array2
are combined into a single array, mergedArray
, using the spread syntax. This method is both concise and efficient, ensuring that your arrays are merged seamlessly without needing to use other methods like concat()
.
Using the Spread Operator in Function Calls
The spread operator can also be used in function calls to handle dynamic argument lists. This is useful when passing zero or more arguments to a function.
Example: Using Spread in Function Calls
function calculateSum(a, b, c) {
return a + b + c
}
const numbers = [1, 2, 3]
const result = calculateSum(...numbers)
In this example, the spread syntax expands the numbers
array into separate arguments for the calculateSum
function. This technique is particularly useful when working with functions that accept a variable number of arguments.
Handling Nested Objects in React State
React often involves managing complex data structures like nested objects. The spread operator simplifies the process of updating specific levels of these objects without mutating the entire object.
Example: Updating Nested Objects
const [user, setUser] = useState({
name: 'Alice',
preferences: { theme: 'light', notifications: true }
})
function updatePreferences(newPreferences) {
setUser({
...user,
preferences: { ...user.preferences, ...newPreferences }
})
}
Here, the preferences
object within user
is updated using the spread operator. This method ensures that only the relevant key value pairs within the nested objects are updated, leaving the rest of the state intact.
Conclusion
The spread operator and spread syntax are indispensable tools in React.js development. They streamline the process of passing props, managing state, and handling arrays and objects within your components. By mastering these techniques, you can write more maintainable and efficient React code that is easier to scale and debug.
Whether you’re managing complex props objects, updating state, or handling function calls, using the spread operator effectively will enhance your ability to develop robust and efficient React applications. For a deeper understanding of the general use of the spread operator in JavaScript, be sure to check out our comprehensive guide on the topic.