How to return multiple values from a JavaScript function
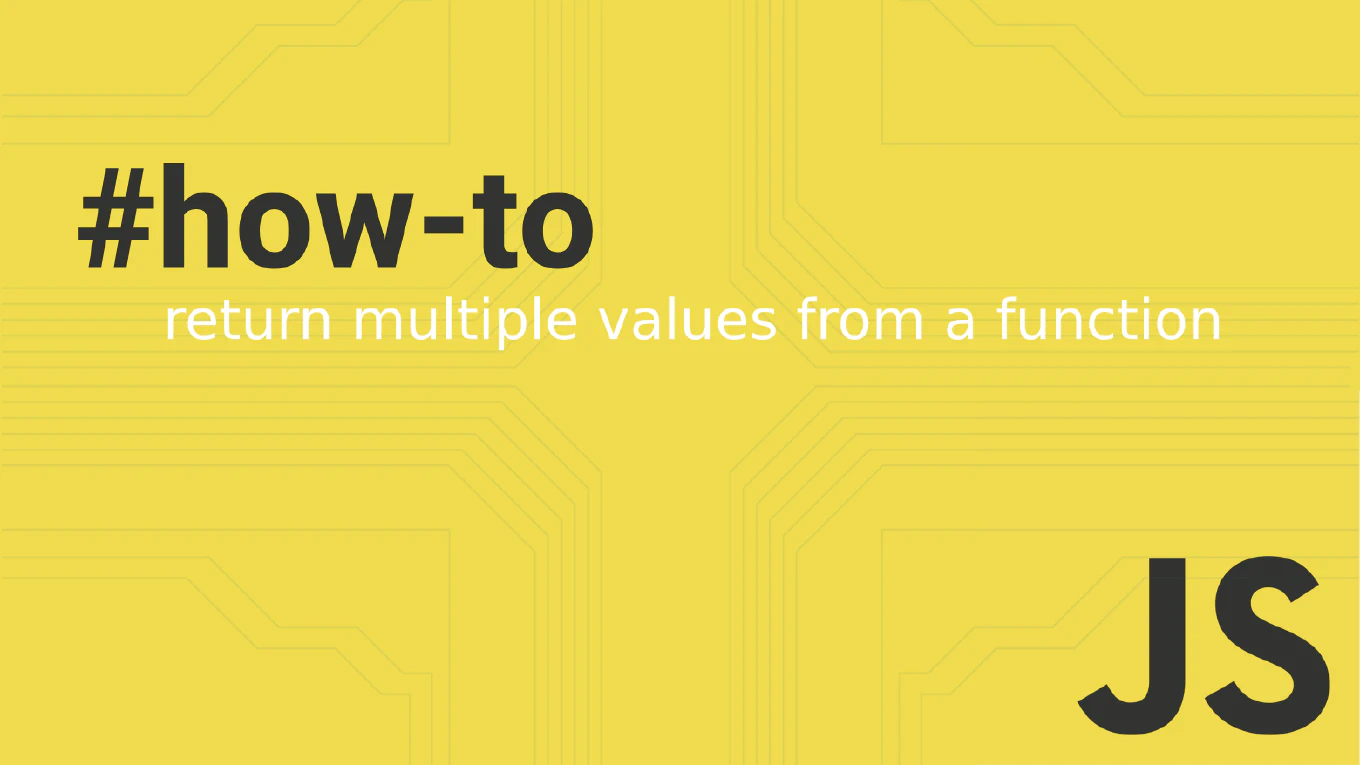
In JavaScript, returning multiple values from a function can be effectively achieved using arrays and objects. This approach allows you to return more than one value from the same javascript function call.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Using Arrays and Array Destructuring
Arrays are a simple way to return multiple values. Here’s an example using array destructuring:
const getCoordinates = () => {
const x = 10
const y = 20
return [x, y]
}
const [x, y] = getCoordinates()
console.log(x) // 10
console.log(y) // 20
In this example, getCoordinates
returns an array with two values, and the destructuring assignment syntax using square brackets is used to assign these values to individual variables.
Using Objects and Key Value Pairs
Using objects allows you to return multiple values with key value pairs, making the returned values more descriptive:
The return value is structured as an object, and you can retrieve the values using object destructuring.
const getPerson = () => {
const firstName = 'Jane'
const lastName = 'Doe'
return { firstName, lastName }
}
const { firstName, lastName } = getPerson()
console.log(firstName) // Jane
console.log(lastName) // Doe
In this example, getPerson
returns an object, and object destructuring is used to extract the individual variables.
Nested Structures
For more complex data, you can nest arrays and objects:
const getUserDetails = () => {
const user = {
name: { first: 'Jane', last: 'Doe' },
age: 28,
address: ['123 Elm St', 'Somewhere', 'USA']
}
return user
}
const { name: { first, last }, age, address: [street, city, country] } = getUserDetails()
console.log(first) // Jane
console.log(last) // Doe
console.log(age) // 28
console.log(street) // 123 Elm St
console.log(city) // Somewhere
console.log(country) // USA
Here, nested destructuring allows extracting multiple values from a complex object structure.
Practical Example
A single function in JavaScript can return multiple values, which is useful for handling and getting more than one value from a function.
Consider a javascript function that processes data and returns multiple computed values:
const processData = (data) => {
const total = data.length
const sum = data.reduce((acc, val) => acc + val, 0)
const average = sum / total
return { total, sum, average }
}
const data = [1, 2, 3, 4, 5]
const { total, sum, average } = processData(data)
console.log(total) // 5
console.log(sum) // 15
console.log(average) // 3
This JavaScript function returns multiple values in an object, demonstrating how to return multiple values from a function in JavaScript.
Conclusion
Returning multiple values from a function in JavaScript is straightforward using arrays and objects. Arrays are suitable for ordered collections of values, while objects provide more clarity with key value pairs. Using destructuring assignment syntax, you can easily extract these values, making your code cleaner and more maintainable. By leveraging these techniques, you can efficiently handle scenarios where more than one value needs to be returned from the same function. Whether you need to return values as a single value, or need to handle multiple values from a function, JavaScript provides flexible and powerful methods to achieve this.