How to validate an email address in JavaScript
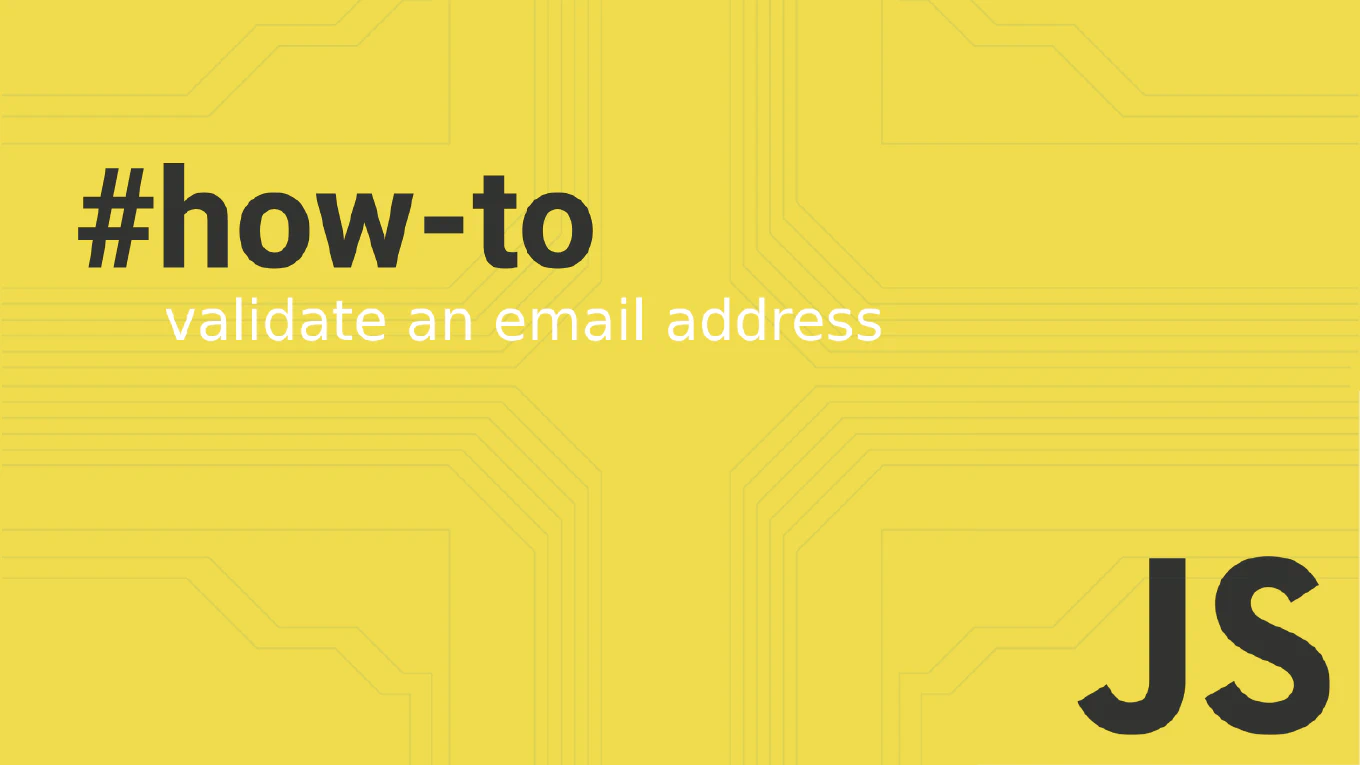
In web development, ensuring that user input is formatted correctly is crucial for maintaining data integrity and enhancing user experience. One common requirement is email validation. Implementing email validation in JavaScript allows developers to provide immediate feedback to users, ensuring that the email addresses entered are valid and correctly formatted. In this guide, we’ll explore how to implement email validation in JavaScript, using regular expressions and other techniques to validate email addresses effectively. We will also provide practical examples and methods using JavaScript code that you can directly copy and paste.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
What Is Email Validation?
Email validation is the process of verifying whether an email address is valid and can receive incoming mail. This involves checking the syntax of the email address to ensure it adheres to standard formats and contains all necessary components, such as the local part, the “@” symbol, and the domain part, including a top-level domain. JavaScript regex for email is commonly used to verify the syntax of email addresses, but comprehensive validation requires more advanced checks to assess the status and risk level of the email address.
Basic Email Validation in JavaScript
A simple way to perform javascript validation for email addresses is by using a basic JavaScript function that checks for the presence of an “@” symbol and at least one dot in the domain part.
const validateEmail = (email) => {
const atSymbol = email.indexOf('@')
const dot = email.lastIndexOf('.')
return atSymbol > 0 && dot > atSymbol
}
This email validation checks if the email address contains the necessary symbols but does not thoroughly verify if the email format is correct or if the email’s domain exists.
Implementing Email Validation with Regular Expressions
For more robust email validation, a regular expression (regex) is used to create a regex pattern that matches valid email formats.
Understanding Regular Expressions
Regular expressions are patterns used for pattern matching within strings. In JavaScript, regex objects are used to define these patterns, and the test
method is applied to check if the input string matches the pattern.
JS email regex is crucial in client-side validation to ensure that only properly formatted email addresses are submitted in forms, enhancing data verification for applications like sign-ups, contact forms, and newsletters.
Crafting a Regex Pattern for Email Validation
An example of a regex pattern for email validation in JavaScript is:
const emailRegex = /^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$/
This pattern checks for:
- Local Part: Lowercase letters (
a-z
), numbers (0-9
), and special characters (.\_%+-
) - "@" Symbol
- Domain Part: Lowercase letters, numbers, and hyphens (
a-z0-9.-
) - Top-Level Domain: At least two lowercase letters (
a-z
)
This regex pattern can be used to validate emails effectively by ensuring the correct format and structure of the email address.
JavaScript Function Using Regex
Here’s how to implement email validation in JavaScript using the regex pattern in your javascript code:
const validateEmail = (email) => {
const emailRegex = /^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$/
return emailRegex.test(email)
}
This validateEmail
function takes an email address as the input string and returns true
if the email is valid according to the regex pattern, or false
otherwise.
Testing Email Addresses
Using the test
method, you can check various email addresses to see if they pass the validation process.
console.log(validateEmail('[email protected]')) // true
console.log(validateEmail('invalid-email')) // false
Common issues that result in invalid email addresses include missing “@” symbols, missing domain parts, or invalid characters in the local part.
Testing email addresses within an HTML form is crucial to provide real-time feedback, ensuring that users input valid email addresses for improved data integrity and user experience.
Advanced Techniques
Server-Side Validation and DNS Lookup
While client-side JavaScript email validation provides immediate feedback, it’s important to perform server-side validation as well. This can include DNS lookups to verify if the email’s domain exists and can receive incoming mail.
Avoiding Poor User Experience
Overly strict email validation can lead to a poor user experience. Ensure that your regex pattern allows for valid characters and accommodates various email formats.
Limitations of Email Validation
While email validation is an essential step in ensuring the quality of user input, it has its limitations. One of the main limitations of email validation is that it only checks the format of the email address, not its existence or validity. This means that an email address can pass the validation check but still be invalid or non-existent. For instance, an email address like “[email protected]” might pass the regex validation but still be undeliverable.
Another limitation of email validation is that it can be bypassed by spammers and bots. Spammers can use techniques such as email address spoofing or email address generation to create fake email addresses that pass the validation check. This highlights the need for additional layers of validation to ensure the authenticity of email addresses.
Alternative Email Validation Methods
In addition to using regex patterns, there are other alternative email validation methods that can be employed. One such method is to use a third-party email validation service. These services leverage advanced algorithms and machine learning techniques to validate email addresses, providing a more accurate result than regex patterns alone.
Another alternative method is to use a DNS lookup to verify the existence of the email address. This method involves checking the DNS records of the domain to see if the email address is valid. By performing a DNS lookup, you can determine whether the domain exists and is capable of receiving emails, adding an extra layer of validation to your process.
Best Practices
- Immediate Feedback: Provide real-time validation in the input field to enhance user experience.
- Form Submission: Validate email addresses during form submission to catch any invalid email addresses before processing.
- Error Messages: Display clear error messages when the email address is not formatted correctly.
Email Validation with CoreUI for React.js
CoreUI is a popular open-source library that provides React components for building modern web applications. Combining React and CoreUI, you can create a user-friendly and responsive form that includes real-time email validation.
Step 1: Setup CoreUI for React.js
To get started, you need to install CoreUI in your React application. Assuming you have a React project set up, install the necessary packages:
npm install @coreui/coreui @coreui/icons @coreui/react
This will install the CoreUI components and icons for use in your project.
Step 2: Create the Email Validation Form
Here, we will build an email validation form using CoreUI’s CForm, CFormInput components, while integrating the email validation logic based on regex.
import React, { useState } from 'react'
import { CForm, CFormInput, CButton } from '@coreui/react'
import '@coreui/coreui/dist/css/coreui.min.css'
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/
const EmailValidationForm = () => {
const [email, setEmail] = useState('')
const [isValid, setIsValid] = useState(null)
const handleEmailChange = (e) => {
const emailInput = e.target.value
setEmail(emailInput)
setIsValid(emailRegex.test(emailInput))
}
const handleSubmit = (e) => {
e.preventDefault()
if (isValid) {
alert('Email is valid!')
} else {
alert('Invalid email address!')
}
}
return (
<CForm onSubmit={handleSubmit}>
<CFormInput
type="email"
id="emailInput"
feedbackInvalid="Email is invalid!"
feedbackValid="Email looks good!"
value={email}
invalid={isValid === false}
valid={isValid === true}
required
onChange={handleEmailChange}
/>
<CButton type="submit" color="primary">Submit</CButton>
</CForm>
)
}
export default EmailValidationForm
Explanation of Key Elements:
- State Management: We use React’s
useState
to track the email input and validation status (isValid
). - Real-time Validation: The
handleEmailChange
function updates the validation status dynamically using the regex pattern when the user types in the input field. - Validation Feedback: CoreUI’s
CFormInput
component provides visual feedback. If the email is valid, it shows a success message; otherwise, it displays an error message. - Form Submission: When the user submits the form, the
handleSubmit
function checks whether the email is valid before proceeding.
Step 3: Run the Application
Once you’ve set up the form, run your React application:
npm start
Your email validation form should now be working, with real-time feedback on email validity based on the regex pattern you provided.
Conclusion
Validating email addresses is a critical step in web development to ensure data integrity and improve email deliverability. By implementing email validation in JavaScript using regular expressions, developers can efficiently verify user input and maintain high-quality data standards.