Passing props to child components in React function components
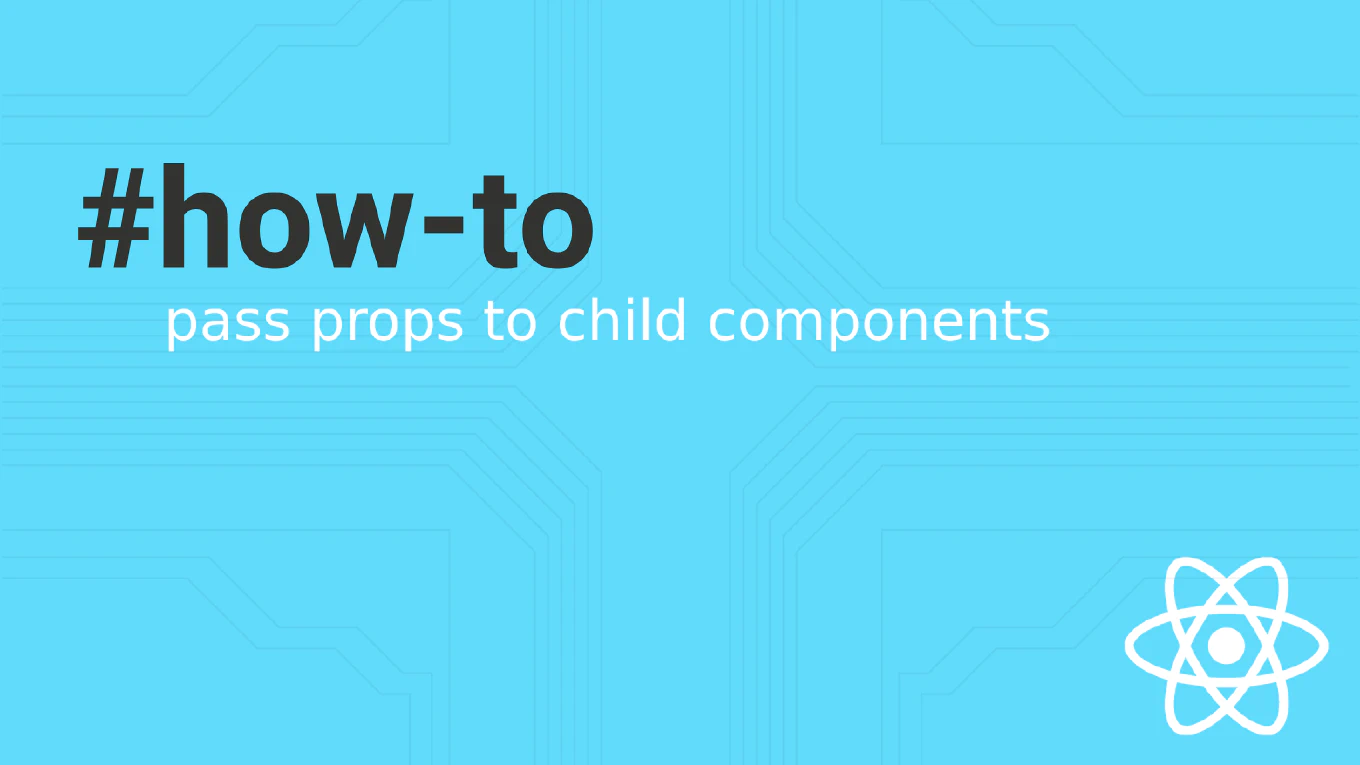
Passing props in React is a fundamental concept that allows data to flow between components. When building user interfaces with React, you often need to pass additional props from a parent component to its child components. This article explores how to pass props to child components, focusing on function components and best practices.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding Parent and Child Components
In React applications, the parent component and child component are core concepts in the React component hierarchy. The parent component is a React component that renders one or more child components. Understanding how to pass data between these components is crucial for building efficient and maintainable code.
Understanding the Children Props
In React, the children
prop is a reserved prop that can be used to pass components or elements as children to a parent component. This allows for the creation of nested structures and the composition of components in a flexible and dynamic manner. The children prop is commonly used when creating reusable and flexible components in React, enabling the construction of complex user interfaces with ease.
It’s useful for component composition, enabling you to create reusable and flexible components.
function ParentComponent({ children }) {
return <div>{children}</div>
}
Here, ParentComponent
renders whatever child elements are passed to it. But how do you pass new props to these child components?
Passing Props to Children Components
There are several methods to pass props to child components:
Using React.cloneElement
The React.cloneElement
function allows you to clone a React element and pass new props to it. This is useful when you have only one child or want to pass additional props to child elements.
function ParentComponent({ children }) {
return React.cloneElement(children, { newProp: 'value' })
}
This function returns a new React element with the merged props, effectively passing new props to the child component.
Mapping Over Children
If you have multiple child components, you can use the Children.map function to iterate over them and pass props individually.
function ParentComponent({ children }) {
return (
<div>
{React.Children.map(children, (child) =>
React.cloneElement(child, { newProp: 'value' })
)}
</div>
)
}
This method allows you to pass props to nested children and handle multiple levels of the component tree.
Example with Carousel Component
These methods are used in our components like the React Carousel component:
...
<div className="carousel-inner">
{Children.map(children, (child, index) => {
if (React.isValidElement(child)) {
return React.cloneElement(child, {
active: active === index,
direction: direction,
key: index,
})
}
return null
})}
</div>
...
Here, the Carousel passes props like active
and direction
to its child components.
Using React’s Context API
The Context API is a popular method for passing data through the component tree without prop drilling. It provides a way to share values like the current theme or authenticated user, which are considered global in a React application.
const MyContext = React.createContext(defaultValue)
function ParentComponent({ children }) {
return (
<MyContext.Provider value={{ data: 'value' }}>
{children}
</MyContext.Provider>
)
}
Child components can consume the context:
function ChildComponent() {
const context = React.useContext(MyContext)
return <div>{context.data}</div>
}
Render Props and Higher-Order Components
Render props and higher-order components (HOCs) are common patterns for passing data to child components.
Render Props
A render prop is a function prop that a component uses to know what to render.
function ParentComponent({ render }) {
return <div>{render()}</div>
}
function App() {
return (
<ParentComponent
render={() => <ChildComponent data="value" />}
/>
)
}
Higher-Order Components
An HOC is a function that takes a component and returns a new component.
function withData(Component) {
return function WrappedComponent(props) {
return <Component {...props} data="value" />
}
}
const EnhancedComponent = withData(ChildComponent)
Practical Example
Let’s see an example of passing props in React:
function ChildComponent({ message }) {
return <div>{message}</div>
}
function ParentComponent() {
const message = 'Hello, World!'
return <ChildComponent message={message} />
}
In this example, the ParentComponent
passes the message prop to the ChildComponent.
Conclusion
Passing props to child components is essential for building user interfaces with React. Whether you’re using component composition, the Context API, or other methods, understanding how to effectively pass data through your component tree is crucial. Remember that the parent component and child component are core concepts in React’s component hierarchy, and mastering the use of children props and React elements will enhance your ability to build complex and efficient React applications.