What is the Difference Between Null and Undefined in JavaScript
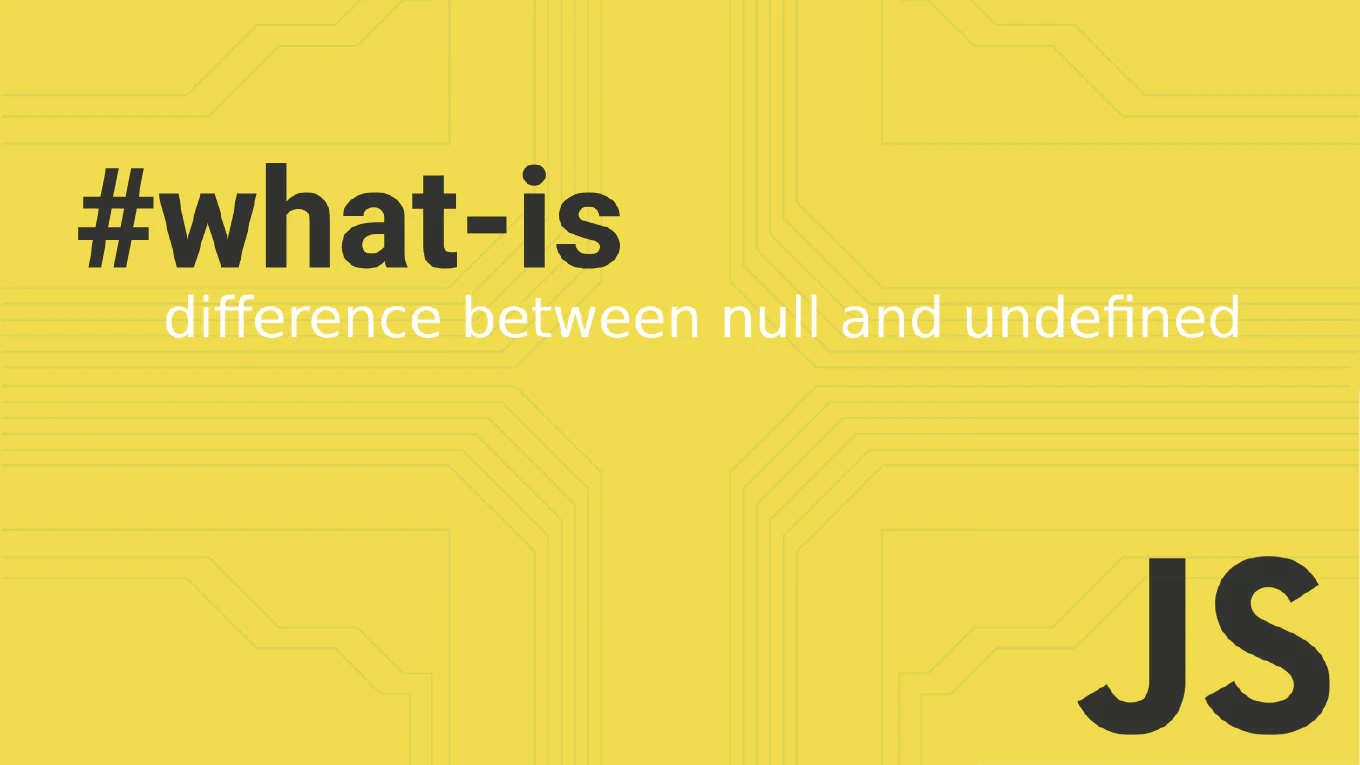
In JavaScript, null
and undefined
are two distinct types representing the absence of a value, but they have different uses and behaviors. This article delves into the differences between null
and undefined
, their use cases, and how to work with them effectively.
What is undefined
?
undefined
is a primitive value automatically assigned to variables that have been declared but not yet assigned a value. It also represents the return value of functions that do not explicitly return a value. Here’s an example:
let unassignedVariable
console.log(unassignedVariable) // Outputs: undefined
const undefinedFunction = () => {}
console.log(undefinedFunction()) // Outputs: undefined
In the original JavaScript implementation, undefined
was introduced to signify the absence of an assigned value. When a variable is declared without an initial value, it is undefined
.
What is null
?
null
is an assignment value that can be explicitly set to a variable to indicate that it is empty or has no meaningful value. It must be explicitly assigned:
let emptyVariable = null
console.log(emptyVariable) // Outputs: null
Unlike undefined
, null
is often used to explicitly indicate the intentional absence of any object value.
Key Differences Between null
and undefined
Type
typeof undefined
returns"undefined"
.
let unassignedVariable
console.log(typeof unassignedVariable) // Outputs: "undefined"
typeof null
returns"object"
due to a bug in the original JavaScript implementation.
let emptyVariable = null
console.log(typeof emptyVariable) // Outputs: "object"
Usage
- Use
undefined
for uninitialized variables or function parameters that have not been assigned a value.
let uninitializedVariable
function logValue(value) {
console.log(value) // Outputs: undefined if no argument is passed
}
logValue(uninitializedVariable)
logValue() // Outputs: undefined
- Use
null
to explicitly indicate that a variable should be empty.
let emptyVariable = null
console.log(emptyVariable) // Outputs: null
function clearValue() {
return null
}
console.log(clearValue()) // Outputs: null
Equality
null
andundefined
are loosely equal (null == undefined
returnstrue
), but not strictly equal (null === undefined
returnsfalse
).
console.log(null == undefined) // Outputs: true
console.log(null === undefined) // Outputs: false
Practical Examples
Consider a scenario where you are dealing with an object that might not have all properties initialized:
const person = {
name: 'Alice',
age: null,
}
console.log(person.age) // Outputs: null
console.log(person.address) // Outputs: undefined
In this example:
person.age
is explicitly assignednull
, indicating that the age is intentionally set to an empty value.person.address
isundefined
because it has not been declared in theperson
object.
Handling null
and undefined
in Functions
When defining functions, you might want to check for both null
and undefined
to ensure robust code:
const checkValue = (value) => {
if (value == null) { // Covers both null and undefined
console.log('Value is either null or undefined')
} else {
console.log('Value is:', value)
}
}
checkValue(null) // Outputs: Value is either null or undefined
checkValue(undefined) // Outputs: Value is either null or undefined
checkValue(42) // Outputs: Value is: 42
Conclusion
Understanding the difference between null
and undefined
is crucial for writing clear and bug-free JavaScript code. Remember:
- Use
undefined
for variables that are declared but not yet assigned a value. - Use
null
to explicitly indicate an empty or non-existent value.
By using these values appropriately, you can make your code more predictable and easier to debug.