How to Use JavaScript setTimeout()
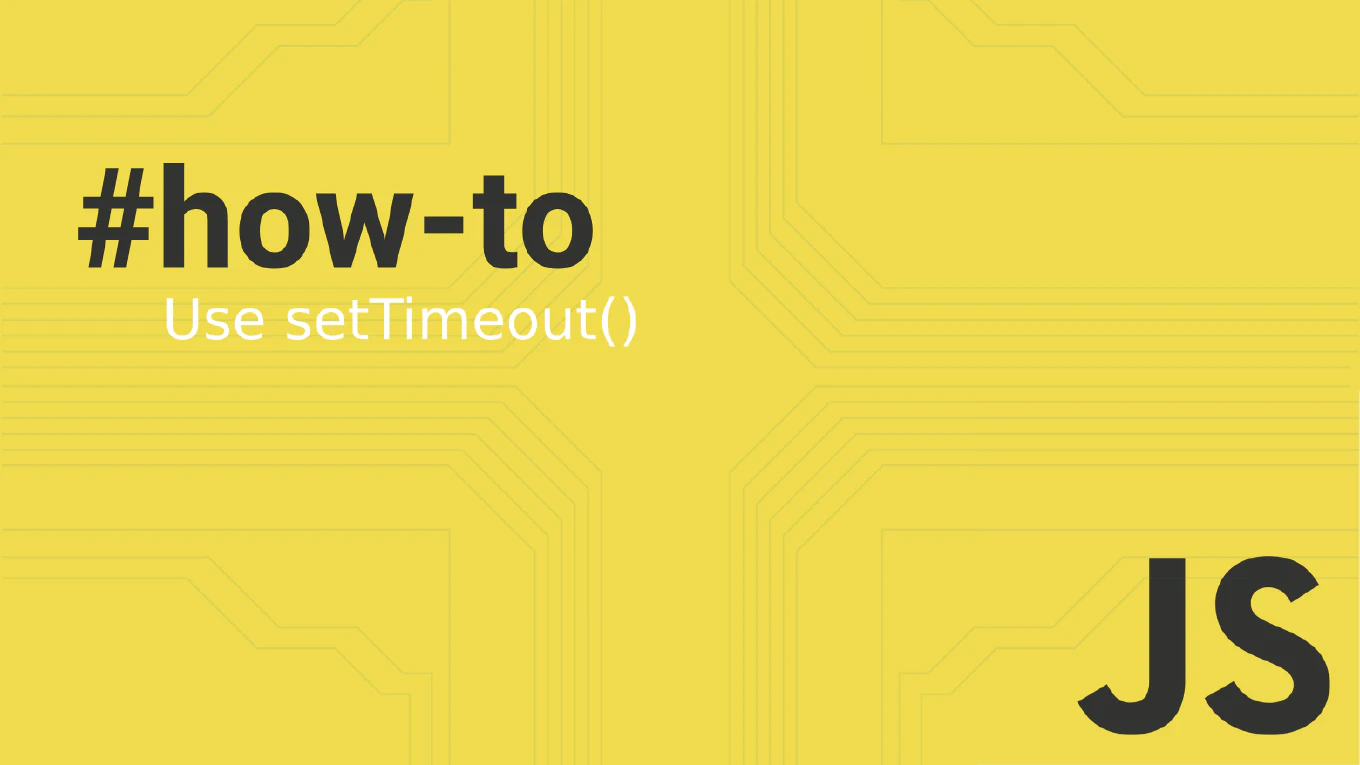
The setTimeout()
function is a fundamental part of JavaScript that allows developers to delay the execution of code. This function is incredibly useful in creating a more interactive and dynamic user experience in web applications by scheduling tasks, managing delays, or even delaying actions within an application. In this blog post, we’ll explore how to use setTimeout()
effectively, ensuring even those new to programming can grasp and implement this powerful feature.
What is setTimeout()?
At its core, setTimeout()
is a method that tells JavaScript to perform a task after a specified period of time. This task can be as simple as displaying a message or updating the state of an application after a delay. The function takes two main arguments: the function to execute and the delay before execution, measured in milliseconds.
Basic Syntax of setTimeout()
The syntax for setTimeout() is straightforward:
const timeoutID = setTimeout(functionToRun, delay)
functionToRun
: This is the callback function thatsetTimeout()
will execute once the specified delay is over.delay
: This is the time in milliseconds that the script should wait before executing the callback function.
An ‘alternative syntax’ for setTimeout()
allows for a string to be used instead of a function, which gets evaluated and executed after the delay. However, using this syntax is discouraged due to security risks akin to those associated with eval().
Simple Example of setTimeout()
Let’s look at the following example to understand how setTimeout()
works using an arrow function for a more concise and cleaner approach:
const greet = () => {
console.log('Hello, user!')
}
setTimeout(greet, 2000)
In this example, the greet
function, defined as an arrow function, will run after 2000 milliseconds (or 2 seconds), and it will print “Hello, user!” to the console, demonstrating the use of arrow functions to pass parameters to setTimeout and handle the ’this’ keyword more effectively.
Why Use setTimeout()?
setTimeout()
is particularly useful in scenarios where you don’t want JavaScript to execute a function immediately. For instance:
- Delaying alerts: Showing a welcome message or offer some seconds after a user has landed on a page.
- Throttling calls: Ensuring that a function does not fire too frequently, which can be crucial for performance, especially when making API calls as the user types in an input field.
Passing Arguments to setTimeout()
You can pass additional parameters to the function called by setTimeout()
right after the delay parameter, allowing you to pass arguments directly to the function that will be executed. This method is particularly useful for when you need to pass additional parameters from the setTimeout()
method rather than directly to the function itself:
const greetUser = (name) => {
console.log(`Hello, ${name}!`)
}
setTimeout(greetUser, 3000, 'Alice')
Here, the string ‘Alice’
is passed as an additional parameter to the greetUser
function, which will execute after 3 seconds.
Canceling a setTimeout()
Sometimes, you might need to cancel a timeout before it has a chance to execute. This is done using the clearTimeout()
method call, which requires the ID returned by setTimeout()
to stop the scheduled function call:
const timeoutID = setTimeout(() => {
console.log('This will not run')
}, 5000)
clearTimeout(timeoutID)
In this case, the message inside the setTimeout()
will never print because we cancel the method call of setTimeout()
right away with clearTimeout()
, effectively preventing the function call from occurring.
Practical Use Case: Debouncing User Input
A common practical use of setTimeout()
in frontend development is debouncing. Debouncing is a technique that aims to limit the rate at which a function is executed. Here is a simple example in the context of handling user input:
let debounceTimeout
const handleInputChange = (event) => {
const value = event.target.value
clearTimeout(debounceTimeout)
debounceTimeout = setTimeout(() => {
console.log(`Processing input: ${value}`)
}, 1000)
}
document.getElementById('input-field').addEventListener('input', handleInputChange)
This ensures that the function does not run on every keystroke but rather waits until the user has stopped typing for 1 second.
Conclusion
setTimeout()
is an essential part of JavaScript that offers the ability to add delays to function executions, enhancing the interactivity and responsiveness of web applications. Understanding how to use setTimeout()
will enable you to create better, more efficient JavaScript code.
Whether you’re creating simple animations, managing API requests, or simply delaying certain actions on your webpage, setTimeout()
provides the tools you need to control timing in a sophisticated manner. Explore it, practice it, and watch as your web applications become more dynamic and user-friendly. Happy coding!
Frequently Asked Questions about JavaScript setTimeout()
What is setTimeout() used for in JavaScript?
setTimeout()
is used to schedule a function to run after a specified amount of time. This is useful for delaying the execution of code until a particular condition is met (like waiting for user interaction), or to schedule tasks that should not interfere with other more critical operations.
How do I stop a setTimeout() from running?
You can stop a setTimeout()
from executing by using clearTimeout(). This function requires the timeout ID returned by setTimeout()
as its parameter. Here’s how you can use it:
const myTimeout = setTimeout(() => {
console.log('This will not run')
}, 3000)
clearTimeout(myTimeout)
Can setTimeout() execute before the time delay?
No, setTimeout()
cannot execute before the specified delay, but the delay parameter only guarantees that your callback will not execute before that time has elapsed. Other queued tasks or operations could delay it further than the specified time.
Does setTimeout() block other JavaScript code from running?
No, setTimeout()
is non-blocking. While it schedules a task to run in the future, allowing for a delay, JavaScript will continue to execute other operations in the meantime. This is a part of JavaScript’s asynchronous behavior.
How accurate is setTimeout()?
The delay set in setTimeout()
indicates a minimum time to wait before executing, not the exact time. Browser task scheduling or heavy JavaScript operations might delay the execution further than expected.
Can setTimeout() be used for animations?
While setTimeout()
can be used for simple animations, requestAnimationFrame() is generally recommended for animations in JavaScript. It provides a more efficient and smoother animation loop by allowing the browser to optimize performance.
What happens if I set the delay to 0?
Setting the delay to 0 milliseconds attempts to run the callback as soon as possible, but after the current script execution finishes and the browser UI thread is free. This can be useful to defer an operation to the stack of messages to be processed next.
How to pass parameters to the specified function called by setTimeout()?
Parameters can be passed after the delay argument in setTimeout()
:
const greet = (name) => {
console.log(`Hello, ${name}!`)
}
setTimeout(greet, 1000, 'Alice') // Outputs: Hello, Alice! after 1 second