How to Manage Date and Time in Specific Timezones Using JavaScript
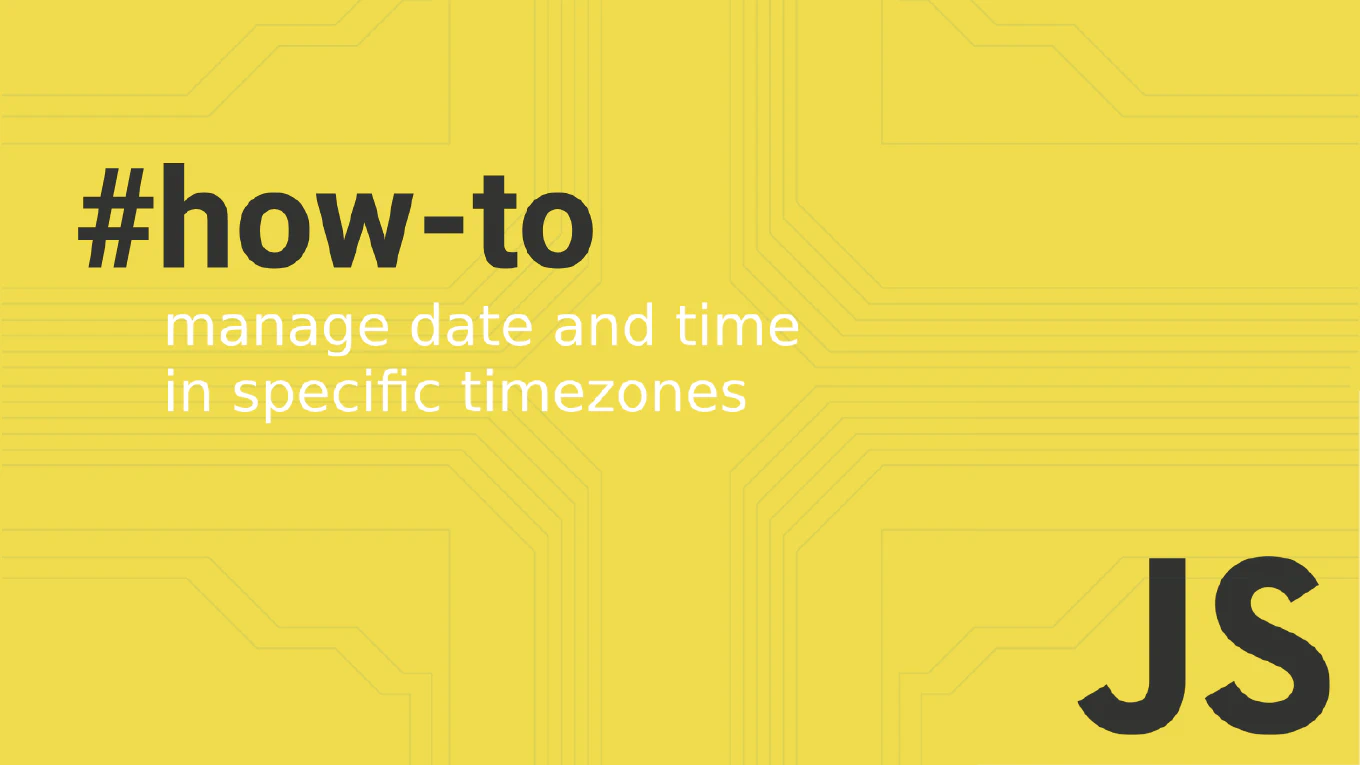
Handling date and time across different timezones is a common yet intricate task for JavaScript developers. Whether you’re working on global applications or time-sensitive services, precise date and time manipulation in specific timezones is essential. JavaScript provides native methods and modern APIs to simplify this task, but challenges like daylight saving time, timezone offsets, and formatting can still cause issues.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
In this guide, you’ll learn how to set, convert, and format JavaScript dates in specific timezones effectively. We’ll explore best practices, modern solutions, and code examples to help you manage dates and times reliably.
Why Timezones Are Tricky in JavaScript
JavaScript’s Date
object is tied closely to the local timezone of the host system. By default, the Date
constructor interprets and outputs time in the system’s local timezone or Coordinated Universal Time (UTC). This default behavior introduces several challenges:
- Timezone Offsets: The difference between UTC and local time can vary due to daylight saving time (DST) changes.
- Consistent Formatting: Applications often need to display dates and times in the user’s timezone or a specified timezone.
- Cross-Timezone Calculations: Performing calculations involving dates in different timezones requires converting between offsets.
- Localization: Formats vary by region, necessitating accurate localization for the desired timezone.
Without proper handling, these issues can lead to bugs and confusion for end users.
Best Practices for Managing Dates and Times in Specific Timezones
1. Using the Intl.DateTimeFormat
API
The Intl.DateTimeFormat
API is a powerful tool for formatting dates and times in specific timezones. It supports IANA timezone identifiers, ensuring accurate formatting even with DST.
const date = new Date() // Current date and time
const formattedDate = new Intl.DateTimeFormat("en-US", {
timeZone: "America/New_York",
dateStyle: "full",
timeStyle: "short",
}).format(date)
console.log(formattedDate) // Example: "Wednesday, January 22, 2025, 5:00 PM"
This approach is ideal for displaying formatted dates without manual timezone offset calculations.
If you’re working with complex calendar components, check out the CoreUI React Calendar or Bootstrap Calendar for prebuilt solutions.
2. Converting Between Timezones Manually
For scenarios where formatting isn’t enough, you may need to manipulate date objects directly. JavaScript’s Date
object provides methods like getTimezoneOffset
to calculate timezone differences.
const utcDate = new Date("2025-01-22T17:00:00Z") // UTC time
const offset = utcDate.getTimezoneOffset() * 60000 // Offset in milliseconds
const localDate = new Date(utcDate.getTime() - offset)
console.log(localDate.toISOString()) // ISO string in local timezone
This method requires careful handling of offsets and doesn’t account for daylight saving time automatically.
3. Using Libraries for Advanced Needs
Libraries like moment-timezone
and date-fns-tz
simplify timezone management. They provide robust tools for creating and converting dates across different timezones.
Example with moment-timezone
:
import moment from "moment-timezone"
const newYorkTime = moment.tz("2025-01-22T17:00:00", "America/New_York")
console.log(newYorkTime.format("YYYY-MM-DD HH:mm:ss")) // "2025-01-22 17:00:00"
Example with date-fns-tz
:
import { zonedTimeToUtc, utcToZonedTime, format } from "date-fns-tz"
const timeZone = "Asia/Tokyo"
const utcDate = zonedTimeToUtc("2025-01-22 17:00:00", timeZone)
const zonedDate = utcToZonedTime(utcDate, timeZone)
console.log(format(zonedDate, "yyyy-MM-dd HH:mm:ssXXX", { timeZone }))
These libraries handle complex cases like daylight saving transitions and support a wide range of timezone data.
For date input and selection in forms, explore the CoreUI React Date Picker or Bootstrap Date Picker for intuitive and customizable solutions.
4. Scheduling Across Timezones
When building global applications, you often need to schedule events for users in different regions. JavaScript’s Intl.DateTimeFormat
API can dynamically adjust for the user’s local timezone.
const meetingTime = new Date("2025-01-22T17:00:00Z") // UTC time
const userTimezone = "Asia/Tokyo" // User's timezone
const localizedTime = new Intl.DateTimeFormat("en-US", {
timeZone: userTimezone,
dateStyle: "medium",
timeStyle: "short",
}).format(meetingTime)
console.log(`Meeting time: ${localizedTime}`) // Example: "Meeting time: Jan 23, 2025, 2:00 AM"
This approach ensures consistency regardless of timezone differences.
Common Issues and Solutions
Daylight Saving Time (DST)
Daylight saving time adjustments can complicate timezone handling. For example, the timezone offset in New York changes during DST transitions. Using APIs like Intl.DateTimeFormat
or libraries ensures these adjustments are handled automatically.
Timezone Abbreviations
Timezone abbreviations (e.g., EST, PST) can cause confusion as they aren’t standardized globally. Relying on IANA timezone names (e.g., America/New_York
) is recommended for accuracy.
Summary
Effectively handling dates and times in specific timezones requires a mix of native JavaScript capabilities and third-party libraries. Here are the key strategies:
- Use the
Intl.DateTimeFormat
API for formatting in specific timezones. - Calculate timezone offsets with
getTimezoneOffset
for manual adjustments. - Rely on libraries like
moment-timezone
ordate-fns-tz
for advanced timezone management. - Test your application thoroughly with diverse timezones and daylight saving transitions.
Next Steps and Resources
To deepen your understanding of timezone handling in JavaScript, consider exploring these resources:
- MDN Web Docs:
Intl.DateTimeFormat
- Moment-Timezone Documentation
- Date-FNS Timezone Module
- IANA Time Zone Database
By applying these best practices and leveraging modern tools, you can ensure accurate and user-friendly timezone handling in your JavaScript applications.