What is Double Question Mark in JavaScript?
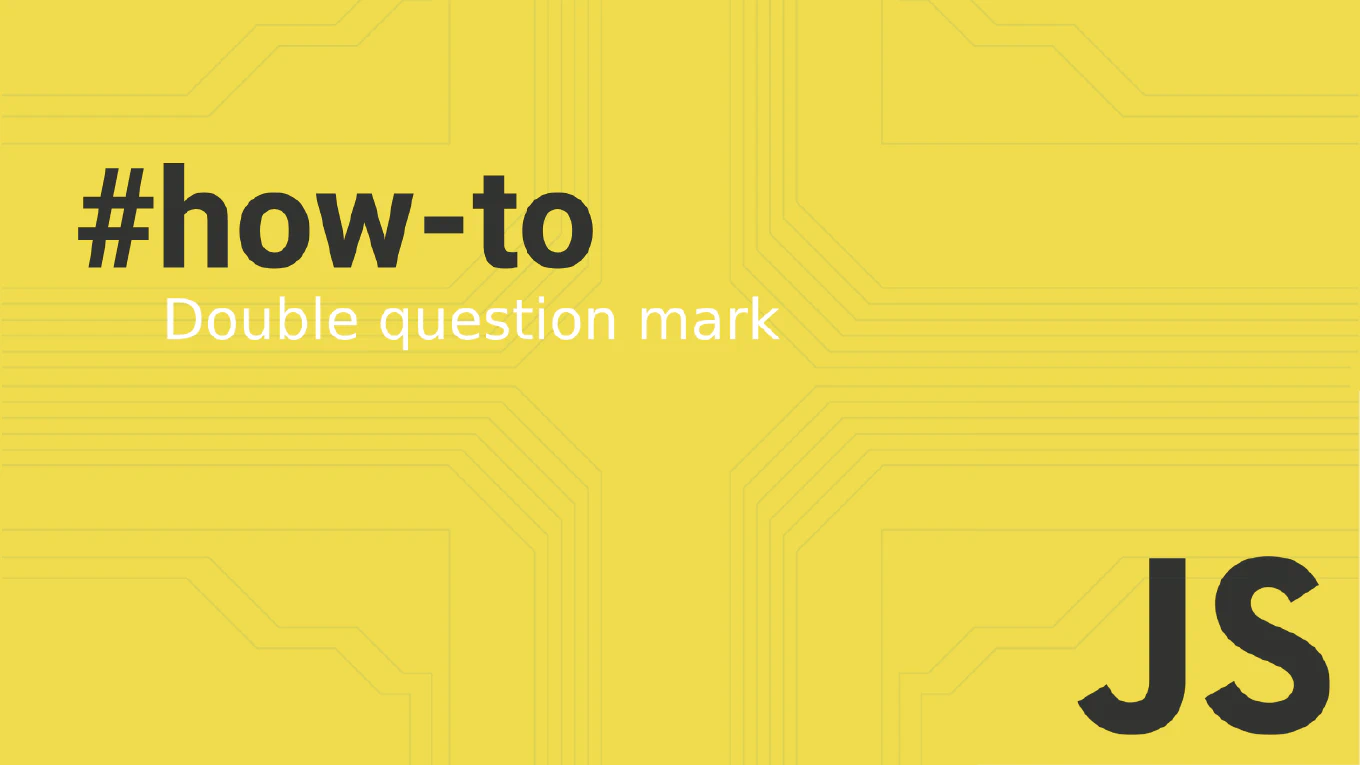
JavaScript is a dynamic programming language rich in features that empower developers to craft interactive and efficient web applications. Among its plethora of tools and operators, the double question mark, or as it’s formally known, the Nullish Coalescing Operator (??
), stands out for its utility and simplicity. This operator, introduced in ECMAScript 2020, is a game-changer for handling variables that might need to be initialized. Let’s dive into this operator’s what, why, and how to understand its significance and application.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
The Essence of Double Question Mark (??)
Imagine you’re creating a personalized greeting on a webpage. You want to use the user’s name if provided; otherwise, you’d default to a friendly “Stranger.” The double question mark operator is your go-to tool for this task. It checks if a value is null or undefined
and, based on that check, either proceeds with that value or opts for an alternative.
Here’s a simple way to visualize it:
const userName = null
const greeting = userName ?? 'Stranger'
console.log(`Hello, ${greeting}`) // "Hello, Stranger"
In this snippet, because userName
is null
, the expression after ?? (in this case, ‘Stranger’) is returned and used for the greeting.
Distinguishing From Other Operators
You might wonder, “Don’t we already use the OR operator (||
) for similar purposes?” While it’s true that the OR operator can serve a similar function, it considers any falsy value (like 0
, false
, an empty string ''
, etc.) as a cue to use the alternative. This is only sometimes desirable. For example:
const userAge = 0
const ageDisplay = userAge || 'Unknown Age'
console.log(`Age: ${ageDisplay}`) // "Age: Unknown Age"
Here, 0
is a valid age, but it is considered falsy and replaced with ‘Unknown Age’ which is not what we intended. The double question mark operator provides precision by only reacting to null
or undefined
, making it a more suitable choice in many cases.
Practical Scenarios and Examples
To truly grasp the versatility and usefulness of the ?? operator, let’s explore some unique, real-world scenarios:
Default Values in User Input
Suppose you’re handling a form where users can input their favorite color. If they leave this field blank, you want to set a default color for them.
const favoriteColor = ''
const displayColor = favoriteColor ?? 'Green'
console.log(`Favorite Color: ${displayColor}`) // "Favorite Color: "
Notice how, unlike with the OR operator, an empty string is considered a valid input and not replaced by ‘Green’. This behavior ensures that user choices, even if seemingly ’empty’, are respected.
Handling Nested Object Properties
When dealing with complex objects, especially those that might have missing properties, the ?? operator can safeguard against undefined errors.
const user = {
profile: {
name: 'Alex'
}
}
const city = user.profile.address?.city ?? 'City not provided'
console.log(city) // "City not provided"
In this example, optional chaining (?.
) safely navigates through the object. If any part of the chain is undefined
, it evaluates to undefined
, and the nullish coalescing operator then provides a default value.
Conclusion
The double question mark in JavaScript is more than just syntactic sugar; it’s a practical tool that enhances code clarity, prevents bugs, and ensures that default values are used only when appropriate. By understanding and applying this operator, beginner developers can write more robust, readable, and efficient JavaScript code. Embrace the power of ?? in your projects and watch your coding practices evolve to new heights.
Frequently Asked Questions
What exactly does the double question mark (??) operator do in JavaScript?
The double question mark, or the Nullish Coalescing Operator, checks if the value to its left is null
or undefined
. If it is, the operator returns the value on its right. Otherwise, it returns the left-hand value. It’s particularly useful for setting default values.
How is the ?? operator different from the OR (||
) operator?
While both operators can be used to provide default values, the key difference lies in their handling of falsy values. The OR (||
) operator considers any falsy value (like 0
, ''
, false
, NaN
) as a cue to return the right-hand value. In contrast, ?? only considers null
or undefined
as triggers for returning the right-hand side value, making it more precise for default assignments.
Can the ?? operator handle multiple conditions?
Yes, you can chain multiple ?? operators together to handle more than two conditions. For example,
const result = value1 ?? value2 ?? 'default'
checks value1 first, then value2, and if both are null
or undefined
, it falls back to ‘default’.
Is it possible to use the ?? operator with non-null/undefined falsy values?
Yes, and that’s where its unique advantage comes into play. For instance, if a value is 0 (which is falsy but not null
or undefined
), the ?? operator will still consider 0
as a valid value and won’t default to the right-hand side value. This behavior is crucial when dealing with numbers, strings, or boolean values where a falsy value (0
, ''
, or false
) is meaningful and should not trigger the use of a default.
Does the ?? operator have any limitations or considerations?
One important consideration is its compatibility with older JavaScript engines that don’t support ES2020 features. Also, when mixing ?? with other logical operators (like || or &&), you should use parentheses to clarify the order of operations and avoid syntax errors or unexpected behaviors. For example, (null || undefined) ?? 'default'
ensures clear and predictable evaluation.
Can I use the ?? operator for default function parameters?
The ?? operator itself can’t directly set default function parameters. However, it can be used inside a function to provide a default value for parameters that are null
or undefined
. For example:
function greet(name) {
const greetingName = name ?? 'Guest'
console.log(`Hello, ${greetingName}`)
}
This setup ensures that greet function uses ‘Guest’ as the default name if none is provided or if the provided name is null
or undefined
.
Is the ?? operator widely supported in JavaScript environments?
As of its introduction in ECMAScript 2020, the ?? operator is supported in most modern browsers and JavaScript environments, including Node.js versions that are based on newer V8 engines. However, for older environments or browsers that do not support ECMAScript 2020 features, a transpiler like Babel may be required to ensure compatibility.
The double question mark operator enriches JavaScript’s syntax by offering a clear and concise way to handle null
and undefined values. Its introduction addresses specific scenarios where developers need precise control over value assignments, emphasizing the language’s continuous evolution towards more expressive and robust coding practices.