What is globalThis in JavaScript?
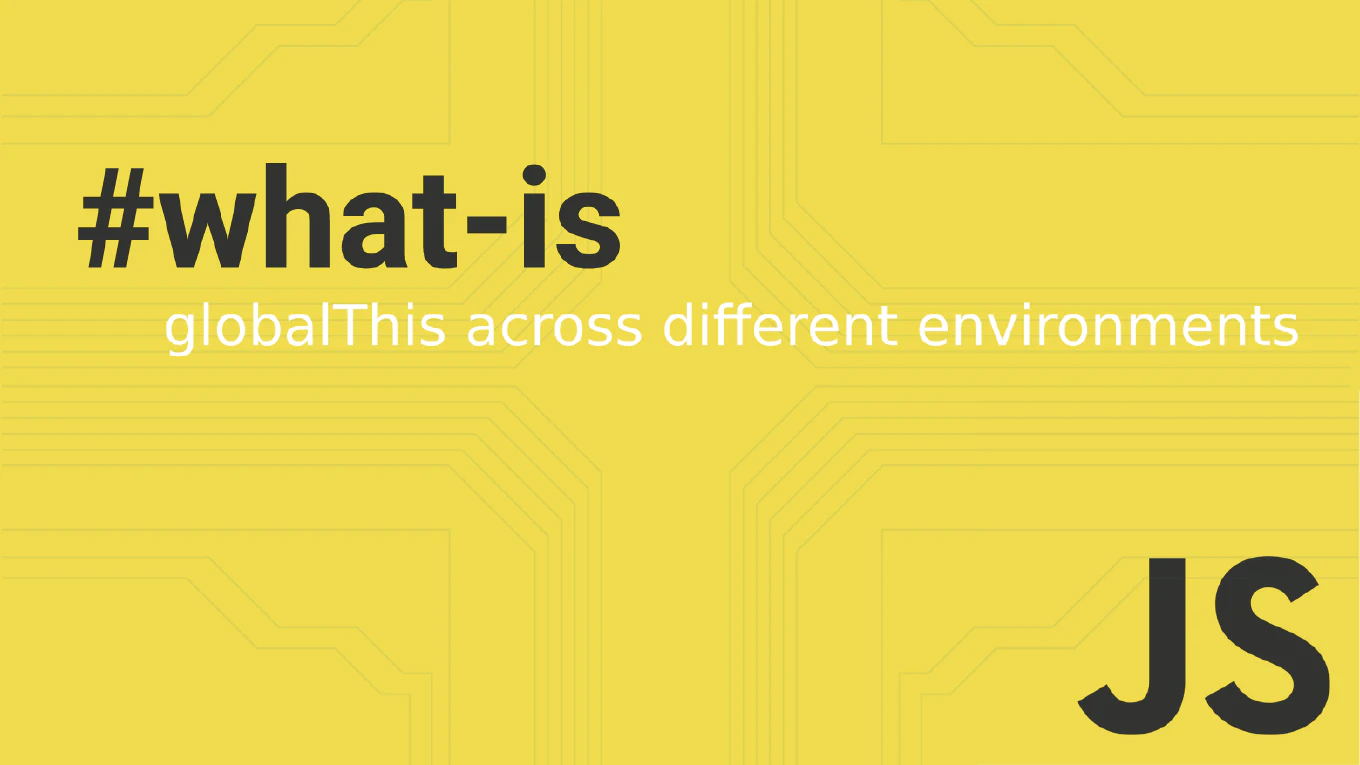
When working with JavaScript, the global object is a critical concept that provides access to the environment’s core functionalities. However, inconsistencies across different JavaScript environments, such as browsers, Node.js, and web workers, have long made accessing the global object challenging. To address this, the globalThis
object was introduced as a unified mechanism to reliably access the global object in a consistent manner, regardless of the environment. This article explores what globalThis
is, why it’s essential, and how to use it effectively.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding the globalThis
Object
The Problem with Previous Approaches
Before globalThis
, accessing the global object depended on the environment:
- In browsers, the global object is the
window
object. - In Node.js, it’s
global
. - In web workers, it’s
self
.
These different global objects required developers to write environment-specific code or use workarounds, such as:
const global = typeof window !== 'undefined' ? window
: typeof global !== 'undefined' ? global
: typeof self !== 'undefined' ? self
: this
This approach was error-prone and cumbersome, especially in non-window contexts or strict mode, where the this
keyword doesn’t behave as expected.
Enter globalThis
The globalThis
object simplifies cross-platform development by providing a unified way to access the global object in all environments. Its value is always the environment’s global object, ensuring consistent behavior.
For example:
console.log(globalThis) // Logs the global object in any JavaScript environment
Key Features of globalThis
- Environment-Agnostic: Works in browsers, Node.js, web workers, and other JavaScript environments.
- Unified Mechanism: Avoids the need for environment-specific syntax.
- Strict Mode Compatibility: Functions seamlessly even in strict mode.
- Standardized Access: Ensures the same value across different environments.
How to Use globalThis
Basic Usage
The globalThis
object provides a standard way to access the global scope:
// Define a global variable
globalThis.myGlobalVariable = 'Accessible globally'
console.log(globalThis.myGlobalVariable) // Output: 'Accessible globally'
Working with Different JavaScript Environments
Whether you’re in a browser, Node.js, or a web worker, globalThis
ensures reliable access:
if (typeof globalThis !== 'undefined') {
console.log('Global object is:', globalThis)
}
Inside Functions
In strict mode, this
inside functions may not refer to the global object. Using globalThis
solves this issue:
'use strict'
function logGlobal() {
console.log(globalThis) // Logs the global object
}
logGlobal()
Example with Web Workers
The globalThis
object is particularly useful in web workers where the window
object is not available:
console.log(globalThis === self) // true in a web worker
Advanced Use Cases
Avoiding Wrong Results
With globalThis
, you can prevent errors caused by different global objects:
// In browsers
console.log(globalThis.window === window) // true
// In Node.js
console.log(typeof globalThis.window) // undefined
Simplifying Code
Using globalThis
can make your code cleaner and more maintainable:
// Instead of checking multiple objects:
const envGlobal = typeof global !== 'undefined' ? global : globalThis
// Use directly:
console.log(globalThis)
Accessing Global Variables
You can define and access global variables without worrying about the specific environment:
globalThis.myGlobal = 42
console.log(globalThis.myGlobal) // 42
Why globalThis
Matters
- Simplifies Cross-Platform Development: Eliminates the need for environment-specific workarounds.
- Consistent Behavior: Provides the same value across multiple environments.
- Future-Proofing Code: Encourages a standard way to interact with the global scope.
- Reduces Errors: Avoids the pitfalls of incorrect global object references in different environments.
Conclusion
The globalThis
object is a small but powerful addition to the JavaScript language, addressing a long-standing inconsistency in accessing the global object. By providing a unified and consistent way to interact with the global scope, it simplifies code, ensures compatibility across environments, and reduces errors. Whether you’re working in a browser, Node.js, or web workers, globalThis
is your go-to tool for accessing the global object.
Next Steps
- Explore the MDN documentation on
globalThis
for more details. - Experiment with
globalThis
in different JavaScript runtimes to understand its behavior fully.