How to Conditionally Add a Property to an Object in JavaScript
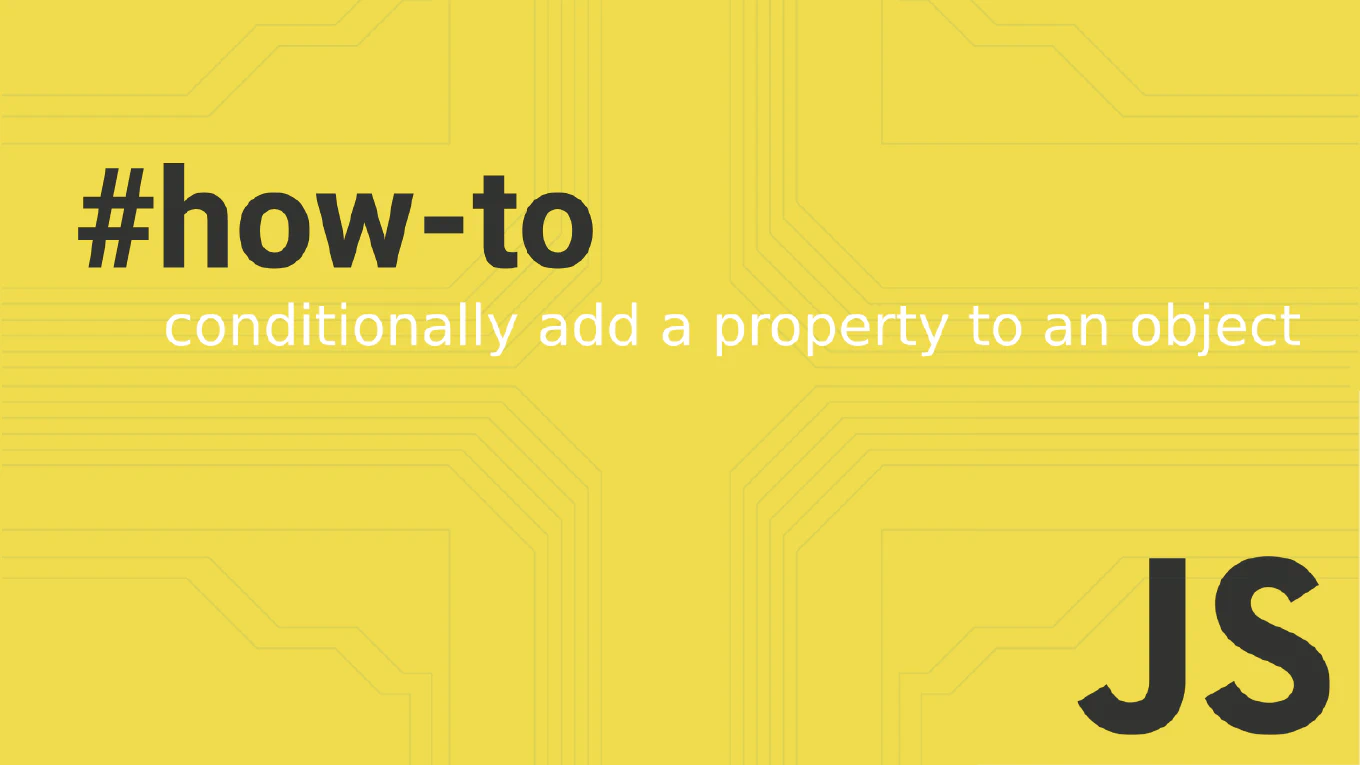
In JavaScript, dynamically adding properties to objects based on conditions is a common task. Whether you’re working with APIs, creating dynamic configurations, or simply managing data, knowing how to conditionally add properties can streamline your code. In this article, we’ll explore different methods to achieve this efficiently, ensuring you write clean, idiomatic code.
Understanding the Basics of Object Creation
JavaScript provides various ways to create objects, such as using object literals, constructors, or factory functions. The most common and concise method is the object literal, which allows you to define a simple object like this:
const person = {
name: 'Alice',
age: 30,
}
However, if you need to conditionally add properties to an object, you’ll need to combine these methods with conditional logic.
Techniques to Conditionally Add Properties
1. Using if
Statements
The straightforward approach is using an if
statement to add properties directly to an object. This method is clear and works well in scenarios where you conditionally add multiple properties.
const person = { name: 'Alice' } // Initial object
const isAdult = true
if (isAdult) {
person.age = 30 // Add property conditionally
}
console.log(person) // Output: { name: 'Alice', age: 30 }
2. Using the Spread Operator
The spread syntax offers a concise way to add properties while creating a new object. This approach avoids mutating the original object, making it ideal for functional programming patterns.
const isAdult = true
const person = {
name: 'Alice',
...(isAdult && { age: 30 }), // Add property if condition is true
}
console.log(person) // Output: { name: 'Alice', age: 30 }
Here, ...(isAdult && { age: 30 })
uses short-circuit evaluation to include the age
property only if isAdult
is true
.
3. Using the Ternary Operator
The ternary operator is another concise way to add properties. You can either modify an existing object or create a new one.
const isEmployed = false
const person = {
name: 'Bob',
age: 25,
job: isEmployed ? 'Engineer' : undefined, // Conditional property
}
console.log(person) // Output: { name: 'Bob', age: 25, job: undefined }
To avoid including properties with undefined
values, you can filter them out later.
4. Combining Logical Operators
You can combine multiple conditions with logical operators for more complex scenarios. This method is efficient when dealing with multiple conditional properties.
const isAdmin = false
const isSubscriber = true
const user = {
username: 'johndoe',
...(isAdmin && { role: 'admin' }),
...(isSubscriber && { subscription: 'pro' }),
}
console.log(user) // Output: { username: 'johndoe', subscription: 'pro' }
Best Practices
- Avoid Mutating Objects: Use spread syntax to create new objects rather than modifying existing ones.
- Filter Undefined Values: Ensure properties with undefined values are removed when they shouldn’t exist.
- Leverage Utility Functions: Encapsulate logic for adding conditional properties in reusable functions.
const addProperty = (obj, key, value, condition) => {
return condition ? { ...obj, [key]: value } : obj
}
const person = { name: 'Alice' }
const updatedPerson = addProperty(person, 'age', 30, true)
console.log(updatedPerson) // Output: { name: 'Alice', age: 30 }
Case Study: Building Dynamic Configurations
Consider an example where you are building server-side configurations. You may want to include certain properties based on the environment.
const isProduction = false
const config = {
apiBaseUrl: 'https://api.example.com',
...(isProduction && { debugMode: false }),
...(!isProduction && { debugMode: true }),
}
console.log(config)
// Output: { apiBaseUrl: 'https://api.example.com', debugMode: true }
This approach ensures you include only relevant properties, keeping your configuration lean.
Summary
By mastering these techniques, you can effectively handle conditional properties in your JavaScript objects. Here’s a quick recap:
- Use if statements for clarity in simple scenarios.
- Leverage the spread operator for concise and functional code.
- Apply the ternary operator for inline conditions.
- Combine logical operators for complex property addition.
- Encapsulate logic in utility functions for reusable solutions.
Next Steps
To further enhance your JavaScript skills, explore these resources:
- MDN Web Docs on Object
- Understanding JavaScript Spread Syntax
- CoreUI Blog: Efficient JavaScript Techniques
By applying these strategies, you’ll write cleaner, more idiomatic code, making your applications both robust and maintainable.