Mastering JavaScript List Comprehension: The Ultimate Guide
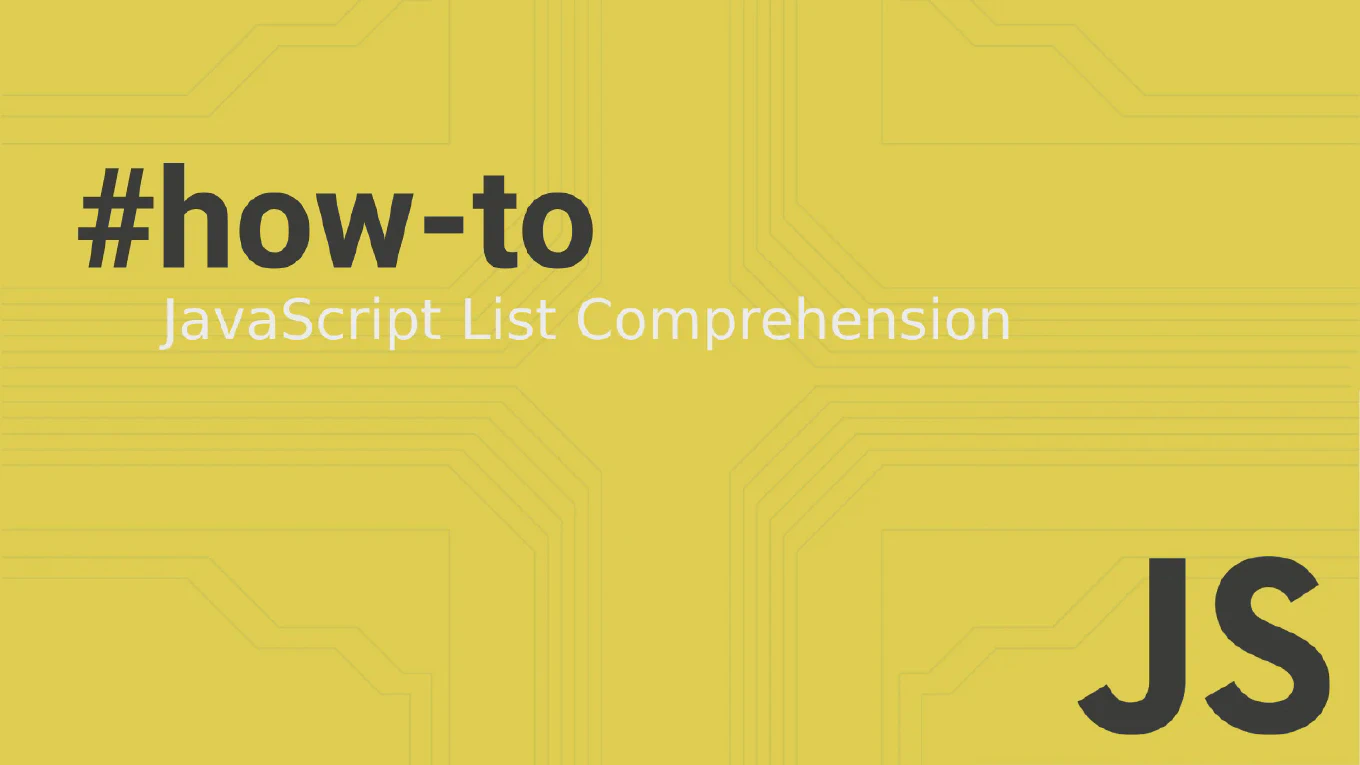
JavaScript list comprehension may not have a dedicated syntax like Python or Perl, but that doesn’t mean it’s any less powerful. In a realm dominated by dynamic data and the need for efficient operations on arrays, understanding how to perform list comprehension in JavaScript is indispensable for developers. This comprehensive guide will walk you through the practical nuances of array manipulation using JavaScript, offering vivid examples to transform you into a master of efficient list handling.
What is list comprehension?
List comprehension is a concise programming technique used to create a new list by applying an operation to each item in an existing iterable. This concept, omnipresent in several programming languages, enables the generation of new lists by filtering and transforming elements swiftly.
Although JavaScript does not provide a built-in list comprehension construct, it offers potent methods to achieve similar outcomes. These include Array.prototype.map()
, Array.prototype.filter()
, and the innovative use of the spread operator (...
). This guide explores the various avenues to achieve list comprehension in JavaScript, ensuring you can confidently tackle any list manipulation task.
Why is it useful in JavaScript?
Array comprehension in JavaScript is a powerful feature that allows for concise and efficient code execution, making it helpful in optimizing performance and creating new arrays from existing ones. It simplifies the process of iterating through arrays, applying conditions and transformations, and generating new arrays in a single line of code. This not only improves the readability of the code but also reduces the overall lines of code, making it easier to maintain and understand.
In JavaScript, methods like Array.prototype.filter()
and Array.prototype.map()
provide similar functionality to Python’s list comprehension. Array.prototype.filter()
allows for easy filtering of elements based on a specific condition. In contrast, Array.prototype.map()
enables the transformation of each element in the array to create a new array.
JavaScript List Comprehension: Array Methods and Techniques
However, this proposition was eventually abandoned, leading to the development of other array methods, such as filter()
and map()
, that are now widely used for manipulating arrays and performing computations in JavaScript.
Transforming Arrays with the Map Method - Array.prototype.map()
The Array.prototype.map()
function shines when you need to transform elements in an array without filtering them out. Let’s dive into several scenarios where map()
becomes indispensable.
Example #1: Create a new array from an existing one
The map()
method is often used to create a new array by applying a function to each element of an existing array.
const numbers = [1, 2, 3, 4, 5]
const doubled = numbers.map(number => number * 2)
console.log(doubled) // Outputs: (5) [2, 4, 6, 8, 10]
Example #2: Rounding Prices
Imagine working with an array of prices and needing only the rounded integer part. The map method, in combination with Math.round()
, makes this task trivial:
const prices = ['$19.99', '$29.99', '$3.45']
const roundedPrices = prices.map(price => `$${Math.round(Number(price.replace('$', '')))}`)
console.log(roundedPrices) // Output: (3) ['$20', '$30', '$3']
Example #3: Extracting a List of Values from a JavaScript Array of Objects
Given an array of objects, extracting specific properties, such as names or countries, becomes a breeze with map()
:
const users = [
{ name: 'Alice', age: 30, country: 'United States' },
{ name: 'Bob', age: 25, country: 'Canada' },
{ name: 'Charlie', age: 35, country: 'United Kingdom' },
{ name: 'Diana', age: 28, country: 'Australia' },
{ name: 'Ethan', age: 22, country: 'New Zealand' }
]
const names = users.map(user => user.name)
console.log(names) // Output: (5) ['Alice', 'Bob', 'Charlie', 'Diana', 'Ethan']
const countries = users.map(user => user.country)
console.log(countries) // Output: (5) ['United States', 'Canada', 'United Kingdom', 'Australia', 'New Zealand']
Example #4: Adding New Values to a JavaScript Array of Objects
You might also want to inject a new property into each object in an array. The map function, used with the spread operator, elegantly accomplishes this:
const cities = [
{ city: 'New York', state: 'New York', postalCode: '10001' },
{ city: 'Los Angeles', state: 'California', postalCode: '90001' },
{ city: 'Chicago', state: 'Illinois', postalCode: '60601' },
{ city: 'Houston', state: 'Texas', postalCode: '77001' },
{ city: 'Phoenix', state: 'Arizona', postalCode: '85001' },
{ city: 'Philadelphia', state: 'Pennsylvania', postalCode: '19101' },
{ city: 'San Antonio', state: 'Texas', postalCode: '78201' },
{ city: 'San Diego', state: 'California', postalCode: '92101' },
{ city: 'Dallas', state: 'Texas', postalCode: '75201' },
{ city: 'San Jose', state: 'California', postalCode: '95101' }
]
const updatedCities = cities.map(city => ({ ...city, country: 'United States' }))
console.log(updatedCities) // Output: (10) [{city: 'New York', state: 'New York', postalCode: '10001', country: 'United States'}, {...}, {...}, ...]
Filtering Arrays with the Filter Method - Array.prototype.filter()
When your task is to create a subset of the original array based on certain conditions, Array.prototype.filter()
is the go-to method.
Example #1: Filter an Array Based on a Condition
Suppose you want to find users with age below 25:
const users = [
{ name: 'Alice', age: 30, country: 'United States' },
{ name: 'Bob', age: 25, country: 'Canada' },
{ name: 'Charlie', age: 35, country: 'United Kingdom' },
{ name: 'Diana', age: 28, country: 'Australia' },
{ name: 'Ethan', age: 22, country: 'New Zealand' }
]
const filteredUsers = users.filter(user => user.age < 25)
console.log(filteredUsers) // Output: (1) [{name: 'Ethan', age: 22, country: 'New Zealand'}]
The Power of Combining Map and Filter
Combining filter()
and map()
unlocks even more significant potential for list manipulation in JavaScript.
After filtering users, you might want to store only names:
const users = [
{ name: 'Alice', age: 30, country: 'United States' },
{ name: 'Bob', age: 25, country: 'Canada' },
{ name: 'Charlie', age: 35, country: 'United Kingdom' },
{ name: 'Diana', age: 28, country: 'Australia' },
{ name: 'Ethan', age: 22, country: 'New Zealand' }
]
const filteredUsers = users.filter(user => user.age < 30)
.map(user => user.name)
console.log(filteredUsers) // Output: (3) ['Bob', 'Diana', 'Ethan']
Iterating with the for…of Statement
The for...of
statement provides another avenue for re-implementing scenarios similar to those achievable with list comprehension:
const users = [
{ name: 'Alice', age: 30, country: 'United States' },
{ name: 'Bob', age: 25, country: 'Canada' },
{ name: 'Charlie', age: 35, country: 'United Kingdom' },
{ name: 'Diana', age: 28, country: 'Australia' },
{ name: 'Ethan', age: 22, country: 'New Zealand' }
]
let filteredUsers = []
for (let user of users) {
if (user.age < 30) {
filteredUsers.push(user.name)
}
}
console.log(filteredUsers) // Output: (3) ['Bob', 'Diana', 'Ethan']
While not a direct counterpart to list comprehension, the for...of
loop and forEach()
method are valuable tools in specific contexts where map()
and filter()
might not suffice.
Conclusion and Takeaways
In JavaScript, list comprehension techniques pivot around understanding and effectively using map()
, filter()
, and the spread operator among other approaches. By mastering these methods, you can elegantly handle array transformations and filtering, making your code more readable and efficient.
Remember, choosing between map()
, filter()
, or a combination thereof depends on your specific needs: transforming data, filtering it, or both. Practice these examples and experiment with them in your projects to gain a deeper understanding and intuition for JavaScript list comprehension.
As you continue to explore and apply these techniques, you’ll recognize their power in making your code functional, elegant, and expressive. Dive in, experiment, and watch your proficiency in JavaScript list comprehension soar to new heights.